mirror of
https://git.proxmox.com/git/fwupd
synced 2025-07-14 20:00:14 +00:00
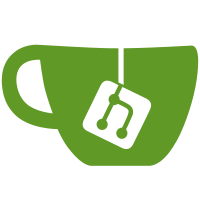
The daemon creates a baseclass of either FuUsbDevice or FuUdevDevice when the devices are added or coldplugged to match the quirk database and to find out what plugin to run. This is proxied to plugins, but they are given the GUsbDevice or GUdevDevice and the FuDevice is just thrown away. Most plugins either use a FuUsbDevice or superclassed version like FuNvmeDevice and so we re-create the FuDevice, re-probe the hardware, re-query the quirk database and then return this to the daemon. In some cases, plugins actually probe the hardware three times (!) by creating a FuUsbDevice to get the quirks, so that the plugin knows what kind of superclass to create, which then itself probes the hardware again. Passing the temporary FuDevice to the plugins means that the simplest ones can just fu_plugin_device_add() the passed in object, or create a superclass and incorporate the actual GUsbDevice and all the GUIDs. This breaks internal plugin API but speeds up startup substantially and deletes a lot of code.
93 lines
2.2 KiB
C
93 lines
2.2 KiB
C
/*
|
|
* Copyright (C) 2016 Richard Hughes <richard@hughsie.com>
|
|
*
|
|
* SPDX-License-Identifier: LGPL-2.1+
|
|
*/
|
|
|
|
#include "config.h"
|
|
|
|
#include "fu-ebitdo-device.h"
|
|
|
|
#include "fu-plugin.h"
|
|
#include "fu-plugin-vfuncs.h"
|
|
|
|
void
|
|
fu_plugin_init (FuPlugin *plugin)
|
|
{
|
|
fu_plugin_add_rule (plugin, FU_PLUGIN_RULE_REQUIRES_QUIRK, FU_QUIRKS_PLUGIN);
|
|
}
|
|
|
|
gboolean
|
|
fu_plugin_usb_device_added (FuPlugin *plugin, FuUsbDevice *device, GError **error)
|
|
{
|
|
g_autoptr(FuDeviceLocker) locker = NULL;
|
|
g_autoptr(FuEbitdoDevice) dev = NULL;
|
|
|
|
/* open the device */
|
|
dev = fu_ebitdo_device_new (device);
|
|
locker = fu_device_locker_new (dev, error);
|
|
if (locker == NULL)
|
|
return FALSE;
|
|
|
|
/* success */
|
|
fu_plugin_device_add (plugin, FU_DEVICE (dev));
|
|
return TRUE;
|
|
}
|
|
|
|
gboolean
|
|
fu_plugin_update (FuPlugin *plugin,
|
|
FuDevice *dev,
|
|
GBytes *blob_fw,
|
|
FwupdInstallFlags flags,
|
|
GError **error)
|
|
{
|
|
GUsbDevice *usb_device = fu_usb_device_get_dev (FU_USB_DEVICE (dev));
|
|
FuEbitdoDevice *ebitdo_dev = FU_EBITDO_DEVICE (dev);
|
|
g_autoptr(FuDeviceLocker) locker = NULL;
|
|
|
|
/* get version */
|
|
if (!fu_device_has_flag (dev, FWUPD_DEVICE_FLAG_IS_BOOTLOADER)) {
|
|
g_set_error_literal (error,
|
|
FWUPD_ERROR,
|
|
FWUPD_ERROR_NOT_SUPPORTED,
|
|
"invalid 8Bitdo device type detected");
|
|
return FALSE;
|
|
}
|
|
|
|
/* write the firmware */
|
|
locker = fu_device_locker_new (ebitdo_dev, error);
|
|
if (locker == NULL)
|
|
return FALSE;
|
|
if (!fu_device_write_firmware (FU_DEVICE (ebitdo_dev), blob_fw, error))
|
|
return FALSE;
|
|
|
|
/* when doing a soft-reboot the device does not re-enumerate properly
|
|
* so manually reboot the GUsbDevice */
|
|
fu_device_set_status (dev, FWUPD_STATUS_DEVICE_RESTART);
|
|
if (!g_usb_device_reset (usb_device, error)) {
|
|
g_prefix_error (error, "failed to force-reset device: ");
|
|
return FALSE;
|
|
}
|
|
|
|
/* wait for replug */
|
|
fu_device_add_flag (dev, FWUPD_DEVICE_FLAG_WAIT_FOR_REPLUG);
|
|
return TRUE;
|
|
}
|
|
|
|
gboolean
|
|
fu_plugin_update_reload (FuPlugin *plugin, FuDevice *dev, GError **error)
|
|
{
|
|
FuEbitdoDevice *ebitdo_dev = FU_EBITDO_DEVICE (dev);
|
|
g_autoptr(FuDeviceLocker) locker = NULL;
|
|
|
|
/* get the new version number */
|
|
locker = fu_device_locker_new (ebitdo_dev, error);
|
|
if (locker == NULL) {
|
|
g_prefix_error (error, "failed to re-open device: ");
|
|
return FALSE;
|
|
}
|
|
|
|
/* success */
|
|
return TRUE;
|
|
}
|