mirror of
https://git.proxmox.com/git/qemu-server
synced 2025-07-02 13:16:36 +00:00
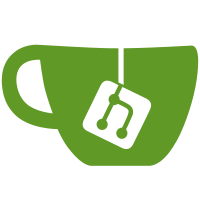
currently if the bridge have a mtu of 9000, when putting a tap interface on it (mtu 1500 by default), the mtu of the bridge goes to 1500. we want that the mtu of the tap interface equal the mtu of the bridge correcting bug: https://bugzilla.proxmox.com/show_bug.cgi?id=258 Signed-off-by: Alexandre Derumier <aderumier@odiso.com>
52 lines
1.3 KiB
Perl
Executable File
52 lines
1.3 KiB
Perl
Executable File
#!/usr/bin/perl -w
|
|
|
|
use strict;
|
|
use PVE::QemuServer;
|
|
use PVE::Tools qw(run_command);
|
|
use PVE::Network;
|
|
|
|
my $iface = shift;
|
|
|
|
die "no interface specified\n" if !$iface;
|
|
|
|
die "got strange interface name '$iface'\n"
|
|
if $iface !~ m/^tap(\d+)i(\d+)$/;
|
|
|
|
my $vmid = $1;
|
|
my $netid = "net$2";
|
|
|
|
my $migratedfrom = $ENV{PVE_MIGRATED_FROM};
|
|
|
|
my $conf = PVE::QemuServer::load_config($vmid, $migratedfrom);
|
|
|
|
die "unable to get network config '$netid'\n"
|
|
if !$conf->{$netid};
|
|
|
|
my $net = PVE::QemuServer::parse_net($conf->{$netid});
|
|
die "unable to parse network config '$netid'\n" if !$net;
|
|
|
|
my $bridge = $net->{bridge};
|
|
die "unable to get bridge setting\n" if !$bridge;
|
|
|
|
my $bridgemtu = PVE::Tools::file_read_firstline("/sys/class/net/$bridge/mtu");
|
|
|
|
system ("/sbin/ifconfig $iface 0.0.0.0 promisc up mtu $bridgemtu") == 0 ||
|
|
die "interface activation failed\n";
|
|
|
|
if ($net->{rate}) {
|
|
|
|
my $debug = 0;
|
|
my $rate = int($net->{rate}*1024*1024);
|
|
my $burst = 1024*1024;
|
|
|
|
PVE::Network::setup_tc_rate_limit($iface, $rate, $burst, $debug);
|
|
}
|
|
|
|
my $newbridge = PVE::Network::activate_bridge_vlan($bridge, $net->{tag});
|
|
PVE::Network::copy_bridge_config($bridge, $newbridge) if $bridge ne $newbridge;
|
|
|
|
system ("/usr/sbin/brctl addif $newbridge $iface") == 0 ||
|
|
die "can't add interface to bridge\n";
|
|
|
|
exit 0;
|