mirror of
https://git.proxmox.com/git/pve-manager
synced 2025-07-15 21:29:28 +00:00
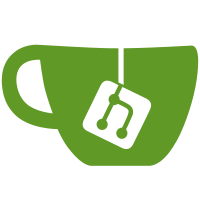
instead of directly setting the minvalue when the maxvalue is lower we invalidate it and only on blur we set the minvalue without this we lose the minvalue when editing only the maxvalue e.g.: Max: 2048 Min: 1024 if we now edit the maxvalue to 4096, on pressing the '4', we are setting the minvalue to '4' because 4 is lower than 1024 Signed-off-by: Dominik Csapak <d.csapak@proxmox.com>
203 lines
4.2 KiB
JavaScript
203 lines
4.2 KiB
JavaScript
Ext.define('PVE.qemu.MemoryInputPanel', {
|
|
extend: 'Proxmox.panel.InputPanel',
|
|
alias: 'widget.pveQemuMemoryPanel',
|
|
onlineHelp: 'qm_memory',
|
|
|
|
insideWizard: false,
|
|
|
|
onGetValues: function(values) {
|
|
var me = this;
|
|
|
|
var res;
|
|
|
|
if (values.memoryType === 'fixed') {
|
|
res = { memory: values.memory };
|
|
if (values.ballooning === '1') {
|
|
// if balloning is active if it is not explicitely set
|
|
res['delete'] = "balloon,shares";
|
|
} else {
|
|
res['delete'] = "shares";
|
|
res.balloon = 0;
|
|
}
|
|
} else {
|
|
res = {
|
|
memory: values.maxmemory,
|
|
balloon: values.balloon
|
|
};
|
|
if (Ext.isDefined(values.shares) && (values.shares !== "")) {
|
|
res.shares = values.shares;
|
|
} else {
|
|
res['delete'] = "shares";
|
|
}
|
|
}
|
|
|
|
return res;
|
|
},
|
|
|
|
initComponent : function() {
|
|
var me = this;
|
|
var labelWidth = 160;
|
|
|
|
var items = [
|
|
{
|
|
xtype: 'radiofield',
|
|
name: 'memoryType',
|
|
inputValue: 'fixed',
|
|
boxLabel: gettext('Use fixed size memory'),
|
|
checked: true,
|
|
listeners: {
|
|
change: function(f, value) {
|
|
if (!me.rendered) {
|
|
return;
|
|
}
|
|
me.down('field[name=memory]').setDisabled(!value);
|
|
me.down('field[name=ballooning]').setDisabled(!value);
|
|
me.down('field[name=maxmemory]').setDisabled(value);
|
|
me.down('field[name=balloon]').setDisabled(value);
|
|
me.down('field[name=shares]').setDisabled(value);
|
|
}
|
|
}
|
|
},
|
|
{
|
|
xtype: 'pveMemoryField',
|
|
name: 'memory',
|
|
hotplug: me.hotplug,
|
|
fieldLabel: gettext('Memory') + ' (MiB)',
|
|
labelAlign: 'right',
|
|
labelWidth: labelWidth
|
|
},
|
|
{
|
|
xtype: 'proxmoxcheckbox',
|
|
hotplug: me.hotplug,
|
|
name: 'ballooning',
|
|
value: '1',
|
|
labelAlign: 'right',
|
|
labelWidth: labelWidth,
|
|
fieldLabel: gettext('Ballooning')
|
|
},
|
|
{
|
|
xtype: 'radiofield',
|
|
name: 'memoryType',
|
|
inputValue: 'dynamic',
|
|
boxLabel: gettext('Automatically allocate memory within this range'),
|
|
listeners: {
|
|
change: function(f, value) {
|
|
if (!me.rendered) {
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
},
|
|
{
|
|
xtype: 'pveMemoryField',
|
|
name: 'maxmemory',
|
|
hotplug: me.hotplug,
|
|
disabled: true,
|
|
value: '1024',
|
|
fieldLabel: gettext('Maximum memory') + ' (MiB)',
|
|
labelAlign: 'right',
|
|
labelWidth: labelWidth,
|
|
listeners: {
|
|
change: function(f, value) {
|
|
var bf = me.down('field[name=balloon]');
|
|
var balloon = bf.getValue();
|
|
bf.setMaxValue(value);
|
|
bf.validate();
|
|
},
|
|
blur: function(f) {
|
|
var value = f.getValue();
|
|
var bf = me.down('field[name=balloon]');
|
|
var balloon = bf.getValue();
|
|
if (balloon > value) {
|
|
bf.setValue(value);
|
|
}
|
|
}
|
|
}
|
|
},
|
|
{
|
|
xtype: 'proxmoxintegerfield',
|
|
name: 'balloon',
|
|
disabled: true,
|
|
minValue: 0,
|
|
maxValue: 512*1024,
|
|
value: '512',
|
|
step: 32,
|
|
fieldLabel: gettext('Minimum memory') + ' (MiB)',
|
|
labelAlign: 'right',
|
|
labelWidth: labelWidth,
|
|
allowBlank: false
|
|
},
|
|
{
|
|
xtype: 'proxmoxintegerfield',
|
|
name: 'shares',
|
|
disabled: true,
|
|
minValue: 0,
|
|
maxValue: 50000,
|
|
value: '',
|
|
step: 10,
|
|
fieldLabel: gettext('Shares'),
|
|
labelAlign: 'right',
|
|
labelWidth: labelWidth,
|
|
allowBlank: true,
|
|
emptyText: Proxmox.Utils.defaultText + ' (1000)',
|
|
submitEmptyText: false
|
|
}
|
|
];
|
|
|
|
if (me.insideWizard) {
|
|
me.column1 = items;
|
|
} else {
|
|
me.items = items;
|
|
}
|
|
|
|
me.callParent();
|
|
}
|
|
});
|
|
|
|
Ext.define('PVE.qemu.MemoryEdit', {
|
|
extend: 'Proxmox.window.Edit',
|
|
|
|
initComponent : function() {
|
|
var me = this;
|
|
|
|
var memoryhotplug;
|
|
if(me.hotplug) {
|
|
Ext.each(me.hotplug.split(','), function(el) {
|
|
if (el === 'memory') {
|
|
memoryhotplug = 1;
|
|
}
|
|
});
|
|
}
|
|
|
|
var ipanel = Ext.create('PVE.qemu.MemoryInputPanel', {
|
|
hotplug: memoryhotplug
|
|
});
|
|
|
|
Ext.apply(me, {
|
|
subject: gettext('Memory'),
|
|
items: [ ipanel ],
|
|
// uncomment the following to use the async configiguration API
|
|
// backgroundDelay: 5,
|
|
width: 400
|
|
});
|
|
|
|
me.callParent();
|
|
|
|
me.load({
|
|
success: function(response, options) {
|
|
var data = response.result.data;
|
|
|
|
var values = {
|
|
memory: data.memory,
|
|
maxmemory: data.memory,
|
|
balloon: data.balloon,
|
|
ballooning: data.balloon === 0 ? '0' : '1',
|
|
shares: data.shares,
|
|
memoryType: data.balloon ? 'dynamic' : 'fixed'
|
|
};
|
|
ipanel.setValues(values);
|
|
}
|
|
});
|
|
}
|
|
});
|