mirror of
https://git.proxmox.com/git/pve-installer
synced 2025-04-28 16:34:37 +00:00
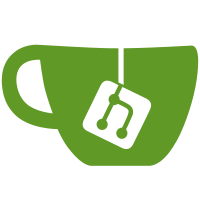
.. such that receivers can differentiate between these two cases more clearly. Sometimes, the `progress` sub does not get passed a text (on purpose!), just updating the progress ratio. This would cause log messages to be written out which could indicate missing text and look rather weird. Signed-off-by: Christoph Heiss <c.heiss@proxmox.com>
87 lines
1.5 KiB
Perl
87 lines
1.5 KiB
Perl
package Proxmox::UI::StdIO;
|
|
|
|
use strict;
|
|
use warnings;
|
|
|
|
use JSON qw(from_json to_json);
|
|
|
|
use base qw(Proxmox::UI::Base);
|
|
|
|
use Proxmox::Log;
|
|
|
|
my sub send_msg {
|
|
my ($type, %values) = @_;
|
|
|
|
my $json = to_json({ type => $type, %values }, { utf8 => 1, canonical => 1 });
|
|
print STDOUT "$json\n";
|
|
}
|
|
|
|
my sub recv_msg {
|
|
my $response = <STDIN> // ''; # FIXME: error handling?
|
|
chomp($response);
|
|
|
|
return eval { from_json($response, { utf8 => 1 }) };
|
|
}
|
|
|
|
sub init {
|
|
my ($self) = @_;
|
|
|
|
STDOUT->autoflush(1);
|
|
}
|
|
|
|
sub message {
|
|
my ($self, $msg) = @_;
|
|
|
|
send_msg('message', message => $msg);
|
|
}
|
|
|
|
sub error {
|
|
my ($self, $msg) = @_;
|
|
|
|
log_error("error: $msg");
|
|
send_msg('error', message => $msg);
|
|
}
|
|
|
|
sub finished {
|
|
my ($self, $success, $msg) = @_;
|
|
|
|
my $state = $success ? 'ok' : 'err';
|
|
log_info("finished: $state, $msg");
|
|
send_msg('finished', state => $state, message => $msg);
|
|
}
|
|
|
|
sub prompt {
|
|
my ($self, $query) = @_;
|
|
|
|
send_msg('prompt', query => $query);
|
|
my $response = recv_msg();
|
|
|
|
if (defined($response) && $response->{type} eq 'prompt-answer') {
|
|
return lc($response->{answer}) eq 'ok';
|
|
}
|
|
}
|
|
|
|
sub display_html {
|
|
my ($raw_html, $html_dir) = @_;
|
|
|
|
log_error("display_html() not available for stdio backend!");
|
|
}
|
|
|
|
sub progress {
|
|
my ($self, $ratio, $text) = @_;
|
|
|
|
if (defined($text)) {
|
|
send_msg('progress', ratio => $ratio, text => $text);
|
|
} else {
|
|
send_msg('progress', ratio => $ratio);
|
|
}
|
|
}
|
|
|
|
sub process_events {
|
|
my ($self) = @_;
|
|
|
|
# nothing to do for now?
|
|
}
|
|
|
|
1;
|