mirror of
https://git.proxmox.com/git/mirror_zfs
synced 2025-04-28 11:40:17 +00:00
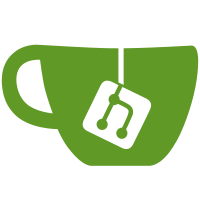
Add a new 'zfs-qemu-packages' GH workflow for manually building RPMs and test installing ZFS RPMs from a yum repo. The workflow has a dropdown menu in the Github runners tab with two options: Build RPMs - Build release RPMs and tarballs and put them into an artifact ZIP file. The directory structure used in the ZIP file mirrors the ZFS yum repo. Test repo - Test install the ZFS RPMs from the ZFS repo. On Almalinux, this will do a DKMS and KMOD test install from both the regular and testing repos. On Fedora, it will do a DKMS install from the regular repo. All test install results will be displayed in the Github runner Summary page. Note that the workflow provides an optional text box where you can specify the full URL to an alternate repo. If left blank, it will install from the default repo from the zfs-release RPM. Most developers will never need to use this workflow. It is intended to be used by the ZFS admins for building and testing releases. This commit also modularizes many of the runner scripts so they can be used by both the zfs-qemu and zfs-qemu-packages workflows. Reviewed-by: Brian Behlendorf <behlendorf1@llnl.gov> Reviewed-by: Tino Reichardt <milky-zfs@mcmilk.de> Signed-off-by: Tony Hutter <hutter2@llnl.gov> Closes #17005
91 lines
2.8 KiB
Bash
Executable File
91 lines
2.8 KiB
Bash
Executable File
#!/bin/bash
|
|
#
|
|
# Do a test install of ZFS from an external repository.
|
|
#
|
|
# USAGE:
|
|
#
|
|
# ./qemu-test-repo-vm [URL]
|
|
#
|
|
# URL: URL to use instead of http://download.zfsonlinux.org
|
|
# If blank, use the default repo from zfs-release RPM.
|
|
|
|
set -e
|
|
|
|
source /etc/os-release
|
|
OS="$ID"
|
|
VERSION="$VERSION_ID"
|
|
|
|
ALTHOST=""
|
|
if [ -n "$1" ] ; then
|
|
ALTHOST="$1"
|
|
fi
|
|
|
|
# Write summary to /tmp/repo so our artifacts scripts pick it up
|
|
mkdir /tmp/repo
|
|
SUMMARY=/tmp/repo/$OS-$VERSION-summary.txt
|
|
|
|
# $1: Repo 'zfs' 'zfs-kmod' 'zfs-testing' 'zfs-testing-kmod'
|
|
# $2: (optional) Alternate host than 'http://download.zfsonlinux.org' to
|
|
# install from. Blank means use default from zfs-release RPM.
|
|
function test_install {
|
|
repo=$1
|
|
host=""
|
|
if [ -n "$2" ] ; then
|
|
host=$2
|
|
fi
|
|
|
|
args="--disablerepo=zfs --enablerepo=$repo"
|
|
|
|
# If we supplied an alternate repo URL, and have not already edited
|
|
# zfs.repo, then update the repo file.
|
|
if [ -n "$host" ] && ! grep -q $host /etc/yum.repos.d/zfs.repo ; then
|
|
sudo sed -i "s;baseurl=http://download.zfsonlinux.org;baseurl=$host;g" /etc/yum.repos.d/zfs.repo
|
|
fi
|
|
|
|
sudo dnf -y install $args zfs zfs-test
|
|
|
|
# Load modules and create a simple pool as a sanity test.
|
|
sudo /usr/share/zfs/zfs.sh -r
|
|
truncate -s 100M /tmp/file
|
|
sudo zpool create tank /tmp/file
|
|
sudo zpool status
|
|
|
|
# Print out repo name, rpm installed (kmod or dkms), and repo URL
|
|
baseurl=$(grep -A 5 "\[$repo\]" /etc/yum.repos.d/zfs.repo | awk -F'=' '/baseurl=/{print $2; exit}')
|
|
package=$(sudo rpm -qa | grep zfs | grep -E 'kmod|dkms')
|
|
|
|
echo "$repo $package $baseurl" >> $SUMMARY
|
|
|
|
sudo zpool destroy tank
|
|
sudo rm /tmp/file
|
|
sudo dnf -y remove zfs
|
|
}
|
|
|
|
echo "##[group]Installing from repo"
|
|
# The openzfs docs are the authoritative instructions for the install. Use
|
|
# the specific version of zfs-release RPM it recommends.
|
|
case $OS in
|
|
almalinux*)
|
|
url='https://raw.githubusercontent.com/openzfs/openzfs-docs/refs/heads/master/docs/Getting%20Started/RHEL-based%20distro/index.rst'
|
|
name=$(curl -Ls $url | grep 'dnf install' | grep -Eo 'zfs-release-[0-9]+-[0-9]+')
|
|
sudo dnf -y install https://zfsonlinux.org/epel/$name$(rpm --eval "%{dist}").noarch.rpm 2>&1
|
|
sudo rpm -qi zfs-release
|
|
test_install zfs $ALTHOST
|
|
test_install zfs-kmod $ALTHOST
|
|
test_install zfs-testing $ALTHOST
|
|
test_install zfs-testing-kmod $ALTHOST
|
|
;;
|
|
fedora*)
|
|
url='https://raw.githubusercontent.com/openzfs/openzfs-docs/refs/heads/master/docs/Getting%20Started/Fedora/index.rst'
|
|
name=$(curl -Ls $url | grep 'dnf install' | grep -Eo 'zfs-release-[0-9]+-[0-9]+')
|
|
sudo dnf -y install -y https://zfsonlinux.org/fedora/$name$(rpm --eval "%{dist}").noarch.rpm
|
|
test_install zfs $ALTHOST
|
|
;;
|
|
esac
|
|
echo "##[endgroup]"
|
|
|
|
# Write out a simple version of the summary here. Later on we will collate all
|
|
# the summaries and put them into a nice table in the workflow Summary page.
|
|
echo "Summary: "
|
|
cat $SUMMARY
|