mirror of
https://git.proxmox.com/git/mirror_novnc
synced 2025-05-02 11:48:11 +00:00
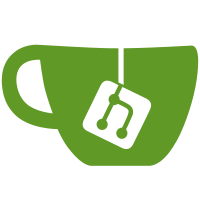
Try to be more consistent in how we capitalize things. Both the "Title Case" and "Sentence case" styles are popular, so either would work. Google and Mozilla both prefer "Sentence case", so let's follow them.
91 lines
3.2 KiB
JavaScript
91 lines
3.2 KiB
JavaScript
import Websock from '../core/websock.js';
|
|
import Display from '../core/display.js';
|
|
|
|
import CopyRectDecoder from '../core/decoders/copyrect.js';
|
|
|
|
import FakeWebSocket from './fake.websocket.js';
|
|
|
|
function testDecodeRect(decoder, x, y, width, height, data, display, depth) {
|
|
let sock;
|
|
let done = false;
|
|
|
|
sock = new Websock;
|
|
sock.open("ws://example.com");
|
|
|
|
sock.on('message', () => {
|
|
done = decoder.decodeRect(x, y, width, height, sock, display, depth);
|
|
});
|
|
|
|
// Empty messages are filtered at multiple layers, so we need to
|
|
// do a direct call
|
|
if (data.length === 0) {
|
|
done = decoder.decodeRect(x, y, width, height, sock, display, depth);
|
|
} else {
|
|
sock._websocket._receiveData(new Uint8Array(data));
|
|
}
|
|
|
|
display.flip();
|
|
|
|
return done;
|
|
}
|
|
|
|
describe('CopyRect decoder', function () {
|
|
let decoder;
|
|
let display;
|
|
|
|
before(FakeWebSocket.replace);
|
|
after(FakeWebSocket.restore);
|
|
|
|
beforeEach(function () {
|
|
decoder = new CopyRectDecoder();
|
|
display = new Display(document.createElement('canvas'));
|
|
display.resize(4, 4);
|
|
});
|
|
|
|
it('should handle the CopyRect encoding', function () {
|
|
// seed some initial data to copy
|
|
display.fillRect(0, 0, 4, 4, [ 0x11, 0x22, 0x33 ]);
|
|
display.fillRect(0, 0, 2, 2, [ 0x00, 0x00, 0xff ]);
|
|
display.fillRect(2, 0, 2, 2, [ 0x00, 0xff, 0x00 ]);
|
|
|
|
let done;
|
|
done = testDecodeRect(decoder, 0, 2, 2, 2,
|
|
[0x00, 0x02, 0x00, 0x00],
|
|
display, 24);
|
|
expect(done).to.be.true;
|
|
done = testDecodeRect(decoder, 2, 2, 2, 2,
|
|
[0x00, 0x00, 0x00, 0x00],
|
|
display, 24);
|
|
expect(done).to.be.true;
|
|
|
|
let targetData = new Uint8Array([
|
|
0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255, 0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255,
|
|
0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255, 0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255,
|
|
0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255, 0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255,
|
|
0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255, 0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255
|
|
]);
|
|
|
|
expect(display).to.have.displayed(targetData);
|
|
});
|
|
|
|
it('should handle empty rects', function () {
|
|
display.fillRect(0, 0, 4, 4, [ 0x00, 0x00, 0xff ]);
|
|
display.fillRect(2, 0, 2, 2, [ 0x00, 0xff, 0x00 ]);
|
|
display.fillRect(0, 2, 2, 2, [ 0x00, 0xff, 0x00 ]);
|
|
|
|
let done = testDecodeRect(decoder, 1, 2, 0, 0,
|
|
[0x00, 0x00, 0x00, 0x00],
|
|
display, 24);
|
|
|
|
let targetData = new Uint8Array([
|
|
0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255, 0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255,
|
|
0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255, 0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255,
|
|
0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255, 0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255,
|
|
0x00, 0xff, 0x00, 255, 0x00, 0xff, 0x00, 255, 0x00, 0x00, 0xff, 255, 0x00, 0x00, 0xff, 255
|
|
]);
|
|
|
|
expect(done).to.be.true;
|
|
expect(display).to.have.displayed(targetData);
|
|
});
|
|
});
|