mirror of
https://git.proxmox.com/git/mirror_corosync
synced 2025-05-24 21:01:29 +00:00
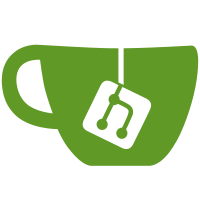
(Logical change 1.98) git-svn-id: http://svn.fedorahosted.org/svn/corosync/trunk@362 fd59a12c-fef9-0310-b244-a6a79926bd2f
154 lines
4.1 KiB
C
154 lines
4.1 KiB
C
/*
|
|
* Copyright (c) 2002-2004 MontaVista Software, Inc.
|
|
*
|
|
* All rights reserved.
|
|
*
|
|
* Author: Steven Dake (sdake@mvista.com)
|
|
*
|
|
* This software licensed under BSD license, the text of which follows:
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* - Redistributions of source code must retain the above copyright notice,
|
|
* this list of conditions and the following disclaimer.
|
|
* - Redistributions in binary form must reproduce the above copyright notice,
|
|
* this list of conditions and the following disclaimer in the documentation
|
|
* and/or other materials provided with the distribution.
|
|
* - Neither the name of the MontaVista Software, Inc. nor the names of its
|
|
* contributors may be used to endorse or promote products derived from this
|
|
* software without specific prior written permission.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
|
|
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
|
|
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
|
|
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
|
|
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
|
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
|
|
* THE POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <assert.h>
|
|
|
|
#include "hdb.h"
|
|
|
|
enum SA_HANDLE_STATE {
|
|
SA_HANDLE_STATE_EMPTY,
|
|
SA_HANDLE_STATE_PENDINGREMOVAL,
|
|
SA_HANDLE_STATE_ACTIVE
|
|
};
|
|
|
|
struct saHandle {
|
|
int state;
|
|
void *instance;
|
|
int refCount;
|
|
};
|
|
|
|
SaErrorT
|
|
saHandleCreate (
|
|
struct saHandleDatabase *handleDatabase,
|
|
int instanceSize,
|
|
unsigned int *handleOut)
|
|
{
|
|
int handle;
|
|
void *newHandles;
|
|
int found = 0;
|
|
void *instance;
|
|
|
|
for (handle = 0; handle < handleDatabase->handleCount; handle++) {
|
|
if (handleDatabase->handles[handle].state == SA_HANDLE_STATE_EMPTY) {
|
|
found = 1;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (found == 0) {
|
|
handleDatabase->handleCount += 1;
|
|
newHandles = (struct saHandle *)realloc (handleDatabase->handles,
|
|
sizeof (struct saHandle) * handleDatabase->handleCount);
|
|
if (newHandles == 0) {
|
|
return (SA_ERR_NO_MEMORY);
|
|
}
|
|
handleDatabase->handles = newHandles;
|
|
}
|
|
|
|
instance = malloc (instanceSize);
|
|
if (instance == 0) {
|
|
return (SA_ERR_NO_MEMORY);
|
|
}
|
|
memset (instance, 0, instanceSize);
|
|
|
|
handleDatabase->handles[handle].state = SA_HANDLE_STATE_ACTIVE;
|
|
|
|
handleDatabase->handles[handle].instance = instance;
|
|
|
|
handleDatabase->handles[handle].refCount = 1;
|
|
|
|
*handleOut = handle;
|
|
|
|
return (SA_OK);
|
|
}
|
|
|
|
|
|
SaErrorT
|
|
saHandleDestroy (
|
|
struct saHandleDatabase *handleDatabase,
|
|
unsigned int handle)
|
|
{
|
|
handleDatabase->handles[handle].state = SA_HANDLE_STATE_PENDINGREMOVAL;
|
|
saHandleInstancePut (handleDatabase, handle);
|
|
|
|
return (SA_OK);
|
|
}
|
|
|
|
|
|
SaErrorT
|
|
saHandleInstanceGet (
|
|
struct saHandleDatabase *handleDatabase,
|
|
unsigned int handle,
|
|
void **instance)
|
|
{
|
|
if (handle >= handleDatabase->handleCount) {
|
|
return (SA_ERR_BAD_HANDLE);
|
|
}
|
|
|
|
if (handleDatabase->handles[handle].state != SA_HANDLE_STATE_ACTIVE) {
|
|
return (SA_ERR_BAD_HANDLE);
|
|
}
|
|
|
|
*instance = handleDatabase->handles[handle].instance;
|
|
|
|
handleDatabase->handles[handle].refCount += 1;
|
|
|
|
return (SA_OK);
|
|
}
|
|
|
|
|
|
SaErrorT
|
|
saHandleInstancePut (
|
|
struct saHandleDatabase *handleDatabase,
|
|
unsigned int handle)
|
|
{
|
|
void *instance;
|
|
|
|
handleDatabase->handles[handle].refCount -= 1;
|
|
assert (handleDatabase->handles[handle].refCount >= 0);
|
|
|
|
if (handleDatabase->handles[handle].refCount == 0) {
|
|
instance = (handleDatabase->handles[handle].instance);
|
|
if (handleDatabase->handleInstanceDestructor) {
|
|
handleDatabase->handleInstanceDestructor (instance);
|
|
}
|
|
free (instance);
|
|
memset (&handleDatabase->handles[handle], 0, sizeof (struct saHandle));
|
|
}
|
|
|
|
return (SA_OK);
|
|
}
|