mirror of
https://git.proxmox.com/git/libgit2
synced 2025-07-02 00:49:27 +00:00
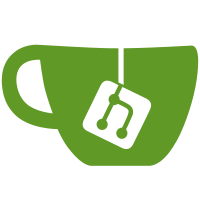
The ownership semantics have been changed all over the library to be consistent. There are no more "borrowed" or duplicated references. Main changes: - `git_repository_open2` and `3` have been dropped. - Added setters and getters to hotswap all the repository owned objects: `git_repository_index` `git_repository_set_index` `git_repository_odb` `git_repository_set_odb` `git_repository_config` `git_repository_set_config` `git_repository_workdir` `git_repository_set_workdir` Now working directories/index files/ODBs and so on can be hot-swapped after creating a repository and between operations. - All these objects now have proper ownership semantics with refcounting: they all require freeing after they are no longer needed (the repository always keeps its internal reference). - Repository open and initialization has been updated to keep in mind the configuration files. Bare repositories are now always detected, and a default config file is created on init. - All the tests affected by these changes have been dropped from the old test suite and ported to the new one.
55 lines
1.6 KiB
C
55 lines
1.6 KiB
C
#include "clay_libgit2.h"
|
|
#include "posix.h"
|
|
|
|
void test_repo_open__bare_empty_repo(void)
|
|
{
|
|
git_repository *repo;
|
|
|
|
cl_git_pass(git_repository_open(&repo, cl_fixture("empty_bare.git")));
|
|
cl_assert(git_repository_path(repo) != NULL);
|
|
cl_assert(git_repository_workdir(repo) == NULL);
|
|
|
|
git_repository_free(repo);
|
|
}
|
|
|
|
void test_repo_open__standard_empty_repo(void)
|
|
{
|
|
git_repository *repo;
|
|
|
|
cl_git_pass(git_repository_open(&repo, cl_fixture("empty_standard_repo/.gitted")));
|
|
cl_assert(git_repository_path(repo) != NULL);
|
|
cl_assert(git_repository_workdir(repo) != NULL);
|
|
|
|
git_repository_free(repo);
|
|
}
|
|
|
|
/* TODO TODO */
|
|
#if 0
|
|
BEGIN_TEST(open2, "Open a bare repository with a relative path escaping out of the current working directory")
|
|
char new_current_workdir[GIT_PATH_MAX];
|
|
char current_workdir[GIT_PATH_MAX];
|
|
char path_repository[GIT_PATH_MAX];
|
|
|
|
const mode_t mode = 0777;
|
|
git_repository* repo;
|
|
|
|
/* Setup the repository to open */
|
|
must_pass(p_getcwd(current_workdir, sizeof(current_workdir)));
|
|
strcpy(path_repository, current_workdir);
|
|
git_path_join_n(path_repository, 3, path_repository, TEMP_REPO_FOLDER, "a/d/e.git");
|
|
must_pass(copydir_recurs(REPOSITORY_FOLDER, path_repository));
|
|
|
|
/* Change the current working directory */
|
|
git_path_join(new_current_workdir, TEMP_REPO_FOLDER, "a/b/c/");
|
|
must_pass(git_futils_mkdir_r(new_current_workdir, mode));
|
|
must_pass(chdir(new_current_workdir));
|
|
|
|
must_pass(git_repository_open(&repo, "../../d/e.git"));
|
|
|
|
git_repository_free(repo);
|
|
|
|
must_pass(chdir(current_workdir));
|
|
must_pass(git_futils_rmdir_r(TEMP_REPO_FOLDER, 1));
|
|
END_TEST
|
|
#endif
|