mirror of
https://git.proxmox.com/git/libgit2
synced 2025-05-08 19:51:31 +00:00
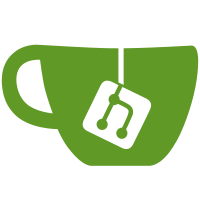
When processing status for a newly checked out repo, it is possible that there will be submodules that have not yet been initialized. The only way to distinguish these from untracked directories is to have some knowledge of submodules. This commit adds a new submodule API which, given a name or path, can determine if it appears to be a submodule and can give information about the submodule.
117 lines
2.5 KiB
C
117 lines
2.5 KiB
C
#include "clar_libgit2.h"
|
|
#include "buffer.h"
|
|
#include "path.h"
|
|
#include "posix.h"
|
|
|
|
static git_repository *g_repo = NULL;
|
|
|
|
void test_status_submodules__initialize(void)
|
|
{
|
|
git_buf modpath = GIT_BUF_INIT;
|
|
|
|
g_repo = cl_git_sandbox_init("submodules");
|
|
|
|
cl_fixture_sandbox("testrepo.git");
|
|
|
|
cl_git_pass(git_buf_sets(&modpath, git_repository_workdir(g_repo)));
|
|
cl_assert(git_path_dirname_r(&modpath, modpath.ptr) >= 0);
|
|
cl_git_pass(git_buf_joinpath(&modpath, modpath.ptr, "testrepo.git\n"));
|
|
|
|
p_rename("submodules/gitmodules", "submodules/.gitmodules");
|
|
cl_git_append2file("submodules/.gitmodules", modpath.ptr);
|
|
|
|
p_rename("submodules/testrepo/.gitted", "submodules/testrepo/.git");
|
|
}
|
|
|
|
void test_status_submodules__cleanup(void)
|
|
{
|
|
cl_git_sandbox_cleanup();
|
|
}
|
|
|
|
void test_status_submodules__api(void)
|
|
{
|
|
git_submodule *sm;
|
|
|
|
cl_assert(git_submodule_lookup(NULL, g_repo, "nonexistent") == GIT_ENOTFOUND);
|
|
|
|
cl_assert(git_submodule_lookup(NULL, g_repo, "modified") == GIT_ENOTFOUND);
|
|
|
|
cl_git_pass(git_submodule_lookup(&sm, g_repo, "testrepo"));
|
|
cl_assert(sm != NULL);
|
|
cl_assert_equal_s("testrepo", sm->name);
|
|
cl_assert_equal_s("testrepo", sm->path);
|
|
}
|
|
|
|
static int
|
|
cb_status__submodule_count(const char *p, unsigned int s, void *payload)
|
|
{
|
|
volatile int *count = (int *)payload;
|
|
|
|
GIT_UNUSED(p);
|
|
GIT_UNUSED(s);
|
|
|
|
(*count)++;
|
|
|
|
return 0;
|
|
}
|
|
|
|
void test_status_submodules__0(void)
|
|
{
|
|
int counts = 0;
|
|
|
|
cl_assert(git_path_isdir("submodules/.git"));
|
|
cl_assert(git_path_isdir("submodules/testrepo/.git"));
|
|
cl_assert(git_path_isfile("submodules/.gitmodules"));
|
|
|
|
cl_git_pass(
|
|
git_status_foreach(g_repo, cb_status__submodule_count, &counts)
|
|
);
|
|
|
|
cl_assert(counts == 6);
|
|
}
|
|
|
|
static const char *expected_files[] = {
|
|
".gitmodules",
|
|
"added",
|
|
"deleted",
|
|
"ignored",
|
|
"modified",
|
|
"untracked"
|
|
};
|
|
|
|
static unsigned int expected_status[] = {
|
|
GIT_STATUS_WT_MODIFIED,
|
|
GIT_STATUS_INDEX_NEW,
|
|
GIT_STATUS_INDEX_DELETED,
|
|
GIT_STATUS_IGNORED,
|
|
GIT_STATUS_WT_MODIFIED,
|
|
GIT_STATUS_WT_NEW
|
|
};
|
|
|
|
static int
|
|
cb_status__match(const char *p, unsigned int s, void *payload)
|
|
{
|
|
volatile int *index = (int *)payload;
|
|
|
|
cl_assert_equal_s(expected_files[*index], p);
|
|
cl_assert(expected_status[*index] == s);
|
|
(*index)++;
|
|
|
|
return 0;
|
|
}
|
|
|
|
void test_status_submodules__1(void)
|
|
{
|
|
int index = 0;
|
|
|
|
cl_assert(git_path_isdir("submodules/.git"));
|
|
cl_assert(git_path_isdir("submodules/testrepo/.git"));
|
|
cl_assert(git_path_isfile("submodules/.gitmodules"));
|
|
|
|
cl_git_pass(
|
|
git_status_foreach(g_repo, cb_status__match, &index)
|
|
);
|
|
|
|
cl_assert(index == 6);
|
|
}
|