mirror of
https://git.proxmox.com/git/libgit2
synced 2025-07-12 09:17:30 +00:00
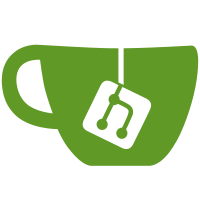
The iterator APIs are not currently consistent with the parameter ordering of the rest of the codebase. This rearranges the order of parameters, simplifies the naming of a number of functions, and makes somewhat better use of macros internally to clean up the iterator code. This also expands the test coverage of iterator functionality, making sure that case sensitive range-limited iteration works correctly.
211 lines
4.9 KiB
C
211 lines
4.9 KiB
C
#include "clar_libgit2.h"
|
|
#include "iterator.h"
|
|
#include "repository.h"
|
|
|
|
static git_repository *g_repo;
|
|
|
|
void test_repo_iterator__initialize(void)
|
|
{
|
|
g_repo = cl_git_sandbox_init("icase");
|
|
}
|
|
|
|
void test_repo_iterator__cleanup(void)
|
|
{
|
|
cl_git_sandbox_cleanup();
|
|
g_repo = NULL;
|
|
}
|
|
|
|
static void expect_iterator_items(
|
|
git_iterator *i, int expected_flat, int expected_total)
|
|
{
|
|
const git_index_entry *entry;
|
|
int count;
|
|
|
|
count = 0;
|
|
cl_git_pass(git_iterator_current(&entry, i));
|
|
|
|
while (entry != NULL) {
|
|
count++;
|
|
|
|
cl_git_pass(git_iterator_advance(&entry, i));
|
|
|
|
if (count > expected_flat)
|
|
break;
|
|
}
|
|
|
|
cl_assert_equal_i(expected_flat, count);
|
|
|
|
cl_git_pass(git_iterator_reset(i, NULL, NULL));
|
|
|
|
count = 0;
|
|
cl_git_pass(git_iterator_current(&entry, i));
|
|
|
|
while (entry != NULL) {
|
|
count++;
|
|
|
|
if (entry->mode == GIT_FILEMODE_TREE)
|
|
cl_git_pass(git_iterator_advance_into(&entry, i));
|
|
else
|
|
cl_git_pass(git_iterator_advance(&entry, i));
|
|
|
|
if (count > expected_total)
|
|
break;
|
|
}
|
|
|
|
cl_assert_equal_i(expected_total, count);
|
|
}
|
|
|
|
/* Index contents (including pseudotrees):
|
|
*
|
|
* 0: a 5: F 10: k/ 16: L/
|
|
* 1: B 6: g 11: k/1 17: L/1
|
|
* 2: c 7: H 12: k/a 18: L/a
|
|
* 3: D 8: i 13: k/B 19: L/B
|
|
* 4: e 9: J 14: k/c 20: L/c
|
|
* 15: k/D 21: L/D
|
|
*
|
|
* 0: B 5: L/ 11: a 16: k/
|
|
* 1: D 6: L/1 12: c 17: k/1
|
|
* 2: F 7: L/B 13: e 18: k/B
|
|
* 3: H 8: L/D 14: g 19: k/D
|
|
* 4: J 9: L/a 15: i 20: k/a
|
|
* 10: L/c 21: k/c
|
|
*/
|
|
|
|
void test_repo_iterator__index(void)
|
|
{
|
|
git_iterator *i;
|
|
git_index *index;
|
|
|
|
cl_git_pass(git_repository_index(&index, g_repo));
|
|
|
|
/* normal index iteration */
|
|
cl_git_pass(git_iterator_for_index(&i, index, 0, NULL, NULL));
|
|
expect_iterator_items(i, 20, 20);
|
|
git_iterator_free(i);
|
|
|
|
git_index_free(index);
|
|
}
|
|
|
|
void test_repo_iterator__index_icase(void)
|
|
{
|
|
git_iterator *i;
|
|
git_index *index;
|
|
unsigned int caps;
|
|
|
|
cl_git_pass(git_repository_index(&index, g_repo));
|
|
caps = git_index_caps(index);
|
|
|
|
/* force case sensitivity */
|
|
cl_git_pass(git_index_set_caps(index, caps & ~GIT_INDEXCAP_IGNORE_CASE));
|
|
|
|
/* normal index iteration with range */
|
|
cl_git_pass(git_iterator_for_index(&i, index, 0, "c", "k/D"));
|
|
expect_iterator_items(i, 7, 7);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_index(&i, index, 0, "k", "k/Z"));
|
|
expect_iterator_items(i, 3, 3);
|
|
git_iterator_free(i);
|
|
|
|
/* force case insensitivity */
|
|
cl_git_pass(git_index_set_caps(index, caps | GIT_INDEXCAP_IGNORE_CASE));
|
|
|
|
/* normal index iteration with range */
|
|
cl_git_pass(git_iterator_for_index(&i, index, 0, "c", "k/D"));
|
|
expect_iterator_items(i, 13, 13);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_index(&i, index, 0, "k", "k/Z"));
|
|
expect_iterator_items(i, 5, 5);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_index_set_caps(index, caps));
|
|
git_index_free(index);
|
|
}
|
|
|
|
void test_repo_iterator__tree(void)
|
|
{
|
|
git_iterator *i;
|
|
git_tree *head;
|
|
|
|
cl_git_pass(git_repository_head_tree(&head, g_repo));
|
|
|
|
/* normal tree iteration */
|
|
cl_git_pass(git_iterator_for_tree(&i, head, 0, NULL, NULL));
|
|
expect_iterator_items(i, 20, 20);
|
|
git_iterator_free(i);
|
|
|
|
git_tree_free(head);
|
|
}
|
|
|
|
void test_repo_iterator__tree_icase(void)
|
|
{
|
|
git_iterator *i;
|
|
git_tree *head;
|
|
git_iterator_flag_t flag;
|
|
|
|
cl_git_pass(git_repository_head_tree(&head, g_repo));
|
|
|
|
flag = GIT_ITERATOR_DONT_IGNORE_CASE;
|
|
|
|
/* normal tree iteration with range */
|
|
cl_git_pass(git_iterator_for_tree(&i, head, flag, "c", "k/D"));
|
|
expect_iterator_items(i, 7, 7);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_tree(&i, head, flag, "k", "k/Z"));
|
|
expect_iterator_items(i, 3, 3);
|
|
git_iterator_free(i);
|
|
|
|
flag = GIT_ITERATOR_IGNORE_CASE;
|
|
|
|
/* normal tree iteration with range */
|
|
cl_git_pass(git_iterator_for_tree(&i, head, flag, "c", "k/D"));
|
|
expect_iterator_items(i, 13, 13);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_tree(&i, head, flag, "k", "k/Z"));
|
|
expect_iterator_items(i, 5, 5);
|
|
git_iterator_free(i);
|
|
}
|
|
|
|
void test_repo_iterator__workdir(void)
|
|
{
|
|
git_iterator *i;
|
|
|
|
/* normal workdir iteration uses explicit tree expansion */
|
|
cl_git_pass(git_iterator_for_workdir(
|
|
&i, g_repo, 0, NULL, NULL));
|
|
expect_iterator_items(i, 12, 22);
|
|
git_iterator_free(i);
|
|
}
|
|
|
|
void test_repo_iterator__workdir_icase(void)
|
|
{
|
|
git_iterator *i;
|
|
git_iterator_flag_t flag;
|
|
|
|
flag = GIT_ITERATOR_DONT_IGNORE_CASE;
|
|
|
|
/* normal workdir iteration with range */
|
|
cl_git_pass(git_iterator_for_workdir(&i, g_repo, flag, "c", "k/D"));
|
|
expect_iterator_items(i, 5, 8);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_workdir(&i, g_repo, flag, "k", "k/Z"));
|
|
expect_iterator_items(i, 1, 4);
|
|
git_iterator_free(i);
|
|
|
|
flag = GIT_ITERATOR_IGNORE_CASE;
|
|
|
|
/* normal workdir iteration with range */
|
|
cl_git_pass(git_iterator_for_workdir(&i, g_repo, flag, "c", "k/D"));
|
|
expect_iterator_items(i, 9, 14);
|
|
git_iterator_free(i);
|
|
|
|
cl_git_pass(git_iterator_for_workdir(&i, g_repo, flag, "k", "k/Z"));
|
|
expect_iterator_items(i, 1, 6);
|
|
git_iterator_free(i);
|
|
}
|