mirror of
https://git.proxmox.com/git/fwupd
synced 2025-07-12 04:17:50 +00:00
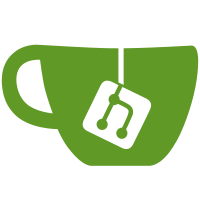
It's actually quite hard to build a front-end for fwupd at the moment as you're never sure when the progress bar is going to zip back to 0% and start all over again. Some plugins go 0..100% for write, others go 0..100% for erase, then again for write, then *again* for verify. By creating a helper object we can easily split up the progress of the specific task, e.g. write_firmware(). We can encode at the plugin level "the erase takes 50% of the time, the write takes 40% and the read takes 10%". This means we can have a progressbar which goes up just once at a consistent speed.
52 lines
1.3 KiB
C
52 lines
1.3 KiB
C
/*
|
|
* Copyright (C) 2015 Richard Hughes <richard@hughsie.com>
|
|
*
|
|
* SPDX-License-Identifier: LGPL-2.1+
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <gusb.h>
|
|
|
|
#include "fu-dfu-device.h"
|
|
#include "fu-dfu-sector.h"
|
|
#include "fu-dfu-target.h"
|
|
|
|
FuDfuTarget *
|
|
fu_dfu_target_new(void);
|
|
|
|
GBytes *
|
|
fu_dfu_target_upload_chunk(FuDfuTarget *self,
|
|
guint16 index,
|
|
gsize buf_sz,
|
|
FuProgress *progress,
|
|
GError **error);
|
|
gboolean
|
|
fu_dfu_target_download_chunk(FuDfuTarget *self,
|
|
guint16 index,
|
|
GBytes *bytes,
|
|
FuProgress *progress,
|
|
GError **error);
|
|
gboolean
|
|
fu_dfu_target_attach(FuDfuTarget *self, FuProgress *progress, GError **error);
|
|
void
|
|
fu_dfu_target_set_alt_idx(FuDfuTarget *self, guint8 alt_idx);
|
|
void
|
|
fu_dfu_target_set_alt_setting(FuDfuTarget *self, guint8 alt_setting);
|
|
|
|
/* for the other implementations */
|
|
void
|
|
fu_dfu_target_set_alt_name(FuDfuTarget *self, const gchar *alt_name);
|
|
void
|
|
fu_dfu_target_set_device(FuDfuTarget *self, FuDfuDevice *device);
|
|
FuDfuDevice *
|
|
fu_dfu_target_get_device(FuDfuTarget *self);
|
|
gboolean
|
|
fu_dfu_target_check_status(FuDfuTarget *self, GError **error);
|
|
FuDfuSector *
|
|
fu_dfu_target_get_sector_for_addr(FuDfuTarget *self, guint32 addr);
|
|
|
|
/* export this just for the self tests */
|
|
gboolean
|
|
fu_dfu_target_parse_sectors(FuDfuTarget *self, const gchar *alt_name, GError **error);
|