mirror of
https://git.proxmox.com/git/fwupd
synced 2025-07-14 20:26:14 +00:00
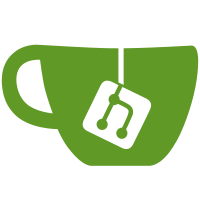
Correctly attach into the alternate mode after the update has completed. The vendor was appending two files to make LVFS distribution 'easier' but I'd much rather use the same deliverables as Windows. This also allows us to simplify the firmware loading.
69 lines
1.4 KiB
C
69 lines
1.4 KiB
C
/*
|
|
* Copyright (C) 2020 Richard Hughes <richard@hughsie.com>
|
|
*
|
|
* SPDX-License-Identifier: LGPL-2.1+
|
|
*/
|
|
|
|
#include "config.h"
|
|
|
|
#include "fu-ccgx-common.h"
|
|
|
|
gchar *
|
|
fu_ccgx_version_to_string (guint32 val)
|
|
{
|
|
/* 16 bits: application type [LSB]
|
|
* 8 bits: build number
|
|
* 4 bits: minor version
|
|
* 4 bits: major version [MSB] */
|
|
return g_strdup_printf ("%u.%u.%u",
|
|
(val >> 28) & 0x0f,
|
|
(val >> 24) & 0x0f,
|
|
(val >> 16) & 0xff);
|
|
}
|
|
|
|
const gchar *
|
|
fu_ccgx_fw_mode_to_string (FWMode val)
|
|
{
|
|
if (val == FW_MODE_BOOT)
|
|
return "BOOT";
|
|
if (val == FW_MODE_FW1)
|
|
return "FW1";
|
|
if (val == FW_MODE_FW2)
|
|
return "FW2";
|
|
return NULL;
|
|
}
|
|
|
|
const gchar *
|
|
fu_ccgx_fw_image_type_to_string (FWImageType val)
|
|
{
|
|
if (val == FW_IMAGE_TYPE_SINGLE)
|
|
return "single";
|
|
if (val == FW_IMAGE_TYPE_DUAL_SYMMETRIC)
|
|
return "dual-symmetric";
|
|
if (val == FW_IMAGE_TYPE_DUAL_ASYMMETRIC)
|
|
return "dual-asymmetric";
|
|
return NULL;
|
|
}
|
|
|
|
FWImageType
|
|
fu_ccgx_fw_image_type_from_string (const gchar *val)
|
|
{
|
|
if (g_strcmp0 (val, "single") == 0)
|
|
return FW_IMAGE_TYPE_SINGLE;
|
|
if (g_strcmp0 (val, "dual-symmetric") == 0)
|
|
return FW_IMAGE_TYPE_DUAL_SYMMETRIC;
|
|
if (g_strcmp0 (val, "dual-asymmetric") == 0)
|
|
return FW_IMAGE_TYPE_DUAL_ASYMMETRIC;
|
|
return FW_IMAGE_TYPE_UNKNOWN;
|
|
}
|
|
|
|
FWMode
|
|
fu_ccgx_fw_mode_get_alternate (FWMode fw_mode)
|
|
{
|
|
if (fw_mode == FW_MODE_FW1)
|
|
return FW_MODE_FW2;
|
|
if (fw_mode == FW_MODE_FW2)
|
|
return FW_MODE_FW1;
|
|
return FW_MODE_BOOT;
|
|
}
|