mirror of
https://git.proxmox.com/git/fwupd
synced 2025-05-03 10:51:03 +00:00
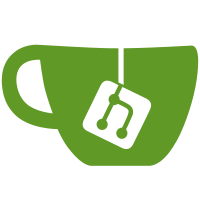
The CustomFlags feature is a bit of a hack where we just join the flags and store in the device metadata section as a string. This makes it inefficient to check if just one flag exists as we have to split the string to a temporary array each time. Rather than adding to the hack by splitting, appending (if not exists) then joining again, store the flags in the plugin privdata directly. This allows us to support negating custom properties (e.g. ~hint) and also allows quirks to append custom values without duplicating them on each GUID match, e.g. [USB\VID_17EF&PID_307F] Plugin = customflag1 [USB\VID_17EF&PID_307F&HUB_0002] Flags = customflag2 ...would result in customflag1,customflag2 which is the same as you'd get from an enumerated device flag doing the same thing.
43 lines
1.5 KiB
C
43 lines
1.5 KiB
C
/*
|
|
* Copyright (C) 2017 Richard Hughes <richard@hughsie.com>
|
|
*
|
|
* SPDX-License-Identifier: LGPL-2.1+
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <fu-device.h>
|
|
#include <xmlb.h>
|
|
|
|
#define fu_device_set_plugin(d,v) fwupd_device_set_plugin(FWUPD_DEVICE(d),v)
|
|
|
|
const gchar *fu_device_internal_flag_to_string (FuDeviceInternalFlags flag);
|
|
FuDeviceInternalFlags fu_device_internal_flag_from_string (const gchar *flag);
|
|
|
|
GPtrArray *fu_device_get_parent_guids (FuDevice *self);
|
|
gboolean fu_device_has_parent_guid (FuDevice *self,
|
|
const gchar *guid);
|
|
GPtrArray *fu_device_get_parent_physical_ids (FuDevice *self);
|
|
gboolean fu_device_has_parent_physical_id (FuDevice *self,
|
|
const gchar *physical_id);
|
|
void fu_device_set_parent (FuDevice *self,
|
|
FuDevice *parent);
|
|
gint fu_device_get_order (FuDevice *self);
|
|
void fu_device_set_order (FuDevice *self,
|
|
gint order);
|
|
void fu_device_set_alternate (FuDevice *self,
|
|
FuDevice *alternate);
|
|
gboolean fu_device_ensure_id (FuDevice *self,
|
|
GError **error)
|
|
G_GNUC_WARN_UNUSED_RESULT;
|
|
void fu_device_incorporate_from_component (FuDevice *device,
|
|
XbNode *component);
|
|
void fu_device_convert_instance_ids (FuDevice *self);
|
|
gchar *fu_device_get_guids_as_str (FuDevice *self);
|
|
GPtrArray *fu_device_get_possible_plugins (FuDevice *self);
|
|
void fu_device_add_possible_plugin (FuDevice *self,
|
|
const gchar *plugin);
|
|
guint64 fu_device_get_private_flags (FuDevice *self);
|
|
void fu_device_set_private_flags (FuDevice *self,
|
|
guint64 flag);
|