mirror of
https://git.proxmox.com/git/fwupd
synced 2025-07-13 08:51:26 +00:00
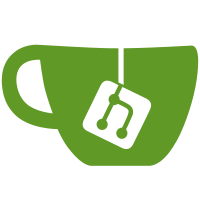
The HSI specification is currently incomplete and in active development. Sample output for my Lenovo P50 Laptop: Host Security ID: HSI:2+UA! HSI-1 ✔ UEFI dbx: OK ✔ TPM: v2.0 ✔ SPI: Write disabled ✔ SPI: Lock enabled ✔ SPI: SMM required ✔ UEFI Secure Boot: Enabled HSI-2 ✔ TPM Reconstruction: Matched PCR0 reading HSI-3 ✘ Linux Kernel S3 Sleep: Deep sleep available HSI-4 ✘ Intel CET: Unavailable Runtime Suffix -U ✔ Firmware Updates: Newest release is 8 months old Runtime Suffix -A ✔ Firmware Attestation: OK Runtime Suffix -! ✔ fwupd plugins: OK ✔ Linux Kernel: OK ✔ Linux Kernel: Locked down ✘ Linux Swap: Not encrypted
55 lines
1.0 KiB
C
55 lines
1.0 KiB
C
/*
|
|
* Copyright (C) 2020 Richard Hughes <richard@hughsie.com>
|
|
*
|
|
* SPDX-License-Identifier: LGPL-2.1+
|
|
*/
|
|
|
|
#include "config.h"
|
|
|
|
#include <string.h>
|
|
|
|
#include "fu-common.h"
|
|
#include "fu-acpi-facp.h"
|
|
|
|
struct _FuAcpiFacp {
|
|
GObject parent_instance;
|
|
gboolean get_s2i;
|
|
};
|
|
|
|
G_DEFINE_TYPE (FuAcpiFacp, fu_acpi_facp, G_TYPE_OBJECT)
|
|
|
|
#define LOW_POWER_S0_IDLE_CAPABLE (1 << 21)
|
|
|
|
FuAcpiFacp *
|
|
fu_acpi_facp_new (GBytes *blob, GError **error)
|
|
{
|
|
FuAcpiFacp *self = g_object_new (FU_TYPE_ACPI_FACP, NULL);
|
|
gsize bufsz = 0;
|
|
guint32 flags = 0;
|
|
const guint8 *buf = g_bytes_get_data (blob, &bufsz);
|
|
|
|
/* parse table */
|
|
if (!fu_common_read_uint32_safe (buf, bufsz, 0x70, &flags, G_LITTLE_ENDIAN, error))
|
|
return FALSE;
|
|
g_debug ("Flags: 0x%04x", flags);
|
|
self->get_s2i = (flags & LOW_POWER_S0_IDLE_CAPABLE) > 0;
|
|
return self;
|
|
}
|
|
|
|
gboolean
|
|
fu_acpi_facp_get_s2i (FuAcpiFacp *self)
|
|
{
|
|
g_return_val_if_fail (FU_IS_ACPI_FACP (self), FALSE);
|
|
return self->get_s2i;
|
|
}
|
|
|
|
static void
|
|
fu_acpi_facp_class_init (FuAcpiFacpClass *klass)
|
|
{
|
|
}
|
|
|
|
static void
|
|
fu_acpi_facp_init (FuAcpiFacp *self)
|
|
{
|
|
}
|