mirror of
https://git.proxmox.com/git/debcargo-conf
synced 2025-04-28 13:24:24 +00:00
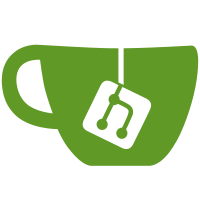
Until yesterday, i had worked in this repo using feature branches like WIP-foo. My practice would be too do work on crate "foo" on a branch WIP-foo off of master, rebase it atop origin/master, and then run ./release.sh there to turn it into pending-foo branch, without having merged the feature into master directly. This change preserves the ability to use that workflow, while not breaking the "do all your work but release on the master branch" for those who prefer it.
152 lines
5.5 KiB
Bash
Executable File
152 lines
5.5 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
. ./vars.sh.frag
|
|
|
|
if test ! -d $PKGDIR_REL; then
|
|
abort 1 "Cannot find $PKGDIR_REL. Did you run ./new-package.sh before?"
|
|
fi
|
|
|
|
if test ! -f "$PKGDIR_REL/debian/changelog"; then
|
|
abort 1 "Cannot find $PKGDIR_REL/debian/changelog. Did you run ./new-package.sh before?"
|
|
fi
|
|
|
|
if git grep --quiet FIXME -- "$PKGDIR_REL" :^"$PKGDIR_REL/debian/*.debcargo.hint" :^"$PKGDIR_REL/debian/changelog"; then
|
|
abort 1 "FIXMEs remain in $PKGDIR_REL, fix and commit those first."
|
|
fi
|
|
|
|
git diff --quiet --cached || \
|
|
abort 1 "You have other pending changes to git, please complete it or stash it away and re-run this script."
|
|
|
|
git diff --quiet -- "$PKGDIR_REL" || \
|
|
abort 1 "Please git-add your changes to $PKGDIR_REL before running"
|
|
|
|
type dch >/dev/null || \
|
|
abort 1 "Install devscripts, we need to run dch."
|
|
|
|
RELBRANCH="pending-$PKGNAME"
|
|
git fetch origin --prune
|
|
|
|
git merge-base --is-ancestor origin/master HEAD || \
|
|
abort 1 "You are not synced with origin/master, please do so before running this script."
|
|
|
|
if head -n1 "$PKGDIR/debian/changelog" | grep -qv UNRELEASED-FIXME-AUTOGENERATED-DEBCARGO; then
|
|
abort 0 "Package already released."
|
|
fi
|
|
|
|
if [ -e "$PKGDIR/debian/BLOCK" ]; then
|
|
abort 1 "TODO items remain in $PKGDIR/debian/BLOCK, please deal with those"
|
|
fi
|
|
|
|
PREVBRANCH="$(git rev-parse --abbrev-ref HEAD)"
|
|
case "$PREVBRANCH" in
|
|
pending-$PKGNAME) true;;
|
|
pending-*) abort 1 "You are on a pending-release branch for a package other than $PKGNAME, $0 can only be run on another branch, like master";;
|
|
*) if git rev-parse -q --verify "refs/heads/$RELBRANCH" >/dev/null || \
|
|
git rev-parse -q --verify "refs/remotes/origin/$RELBRANCH" >/dev/null; then
|
|
git checkout "$RELBRANCH"
|
|
else
|
|
git checkout -b "$RELBRANCH"
|
|
fi;;
|
|
esac
|
|
|
|
if head -n1 "$PKGDIR/debian/changelog" | grep -qv UNRELEASED-FIXME-AUTOGENERATED-DEBCARGO; then
|
|
git checkout "$PREVBRANCH"
|
|
abort 0 "Package already released on branch $RELBRANCH. If that was a mistake then run:\ngit branch -D $RELBRANCH\nAnd re-run this script ($0 $*). You might have to delete the remote branch too:\ngit push --delete origin $RELBRANCH"
|
|
fi
|
|
|
|
( cd "$PKGDIR"
|
|
sed -i -e s/UNRELEASED-FIXME-AUTOGENERATED-DEBCARGO/UNRELEASED/ debian/changelog
|
|
if test -z "$DISTRO"; then
|
|
# To upload to other distro like experimental
|
|
DISTRO=unstable
|
|
fi
|
|
dch -m -r -D $DISTRO ""
|
|
git add debian/changelog
|
|
git rm --ignore-unmatch debian/RFS
|
|
)
|
|
|
|
revert_git_changes() {
|
|
git reset --merge
|
|
git checkout -- "$PKGDIR/debian/changelog"
|
|
git checkout -q -- "$PKGDIR/debian/RFS" || true
|
|
git checkout "$PREVBRANCH"
|
|
git branch -d "$RELBRANCH"
|
|
}
|
|
|
|
if ! run_debcargo --changelog-ready; then
|
|
revert_git_changes
|
|
abort 1 "Release attempt failed to run debcargo, probably the package needs updating (./update.sh $*)"
|
|
fi
|
|
|
|
if ! git diff --exit-code -- "$PKGDIR_REL"; then
|
|
revert_git_changes
|
|
abort 1 "Release attempt resulted in git diffs to $PKGDIR_REL, probably the package needs updating (./update.sh $*)"
|
|
fi
|
|
|
|
if ! ( cd build && SOURCEONLY=1 ./build.sh "$CRATE" $VER ); then
|
|
revert_git_changes
|
|
abort 1 "Release attempt failed (see messages above), possible reasons are: " \
|
|
"- build-dependencies not in Debian => release those first." \
|
|
"- packaged version is out-of-date => run \`./update.sh $*\`"
|
|
fi
|
|
|
|
git commit -m "Release package $PKGNAME"
|
|
|
|
DEBVER=$(dpkg-parsechangelog -l $BUILDDIR/debian/changelog -SVersion)
|
|
DEBSRC=$(dpkg-parsechangelog -l $BUILDDIR/debian/changelog -SSource)
|
|
DEB_HOST_ARCH=$(dpkg-architecture -q DEB_HOST_ARCH)
|
|
cat >&2 <<eof
|
|
Release of $CRATE ready as a source package in ${BUILDDIR#$PWD/}. You need to
|
|
perform the following steps:
|
|
|
|
Build the package if necessary, and upload
|
|
==========================================
|
|
|
|
If the source package is already in Debian and this version does not introduce
|
|
new binaries, then you can just go ahead and directly dput the source package.
|
|
|
|
cd build && dput ${DEBSRC}_${DEBVER}_source.changes
|
|
|
|
For your reference, this source package builds $(grep ^Package build/${CRATE//_/-}/debian/control | wc -l) binary package(s).
|
|
|
|
If this is a NEW source package or introduces NEW binary packages not already
|
|
in the Debian archive, you will need to build a binary package out of it. The
|
|
recommended way is to run something like:
|
|
|
|
cd build && ./build.sh $CRATE $VER && dput ${DEBSRC}_${DEBVER}_${DEB_HOST_ARCH}.changes
|
|
|
|
This assumes you followed the "DD instructions" in README.rst, for setting up
|
|
a build environment for release.
|
|
|
|
If the build fails e.g. due to missing Build-Dependencies you should revert
|
|
what I did (see below) and package those missing Build-Dependencies first.
|
|
|
|
Push this pending-release branch
|
|
================================
|
|
|
|
After you have uploaded the package with dput(1), you should push $RELBRANCH so
|
|
that other people see it's been uploaded. Then, checkout another branch like
|
|
master to continue development on other packages.
|
|
|
|
git push origin $RELBRANCH && git checkout master
|
|
|
|
Merge the pending-release branch when ACCEPTED
|
|
==============================================
|
|
|
|
When it's ACCEPTED by the Debian FTP masters, you may then merge this branch
|
|
back into the master branch, delete it, and push these updates to origin.
|
|
|
|
git checkout master && git merge $RELBRANCH && git branch -d $RELBRANCH
|
|
git push origin master :$RELBRANCH
|
|
|
|
----
|
|
|
|
The above assumes you are a Debian Developer with upload rights. If not, you
|
|
should revert what I just did. To do that, run:
|
|
|
|
git checkout master && git branch -D $RELBRANCH
|
|
|
|
Then ask a Debian Developer to re-run me ($0 $*) on your behalf. Also add your
|
|
crate to the "Ready for upload" list in TODO.rst so it's easy to track.
|
|
eof
|