mirror of
https://github.com/nodejs/node.git
synced 2025-05-02 03:31:35 +00:00
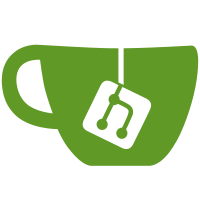
This updates `assert.rejects()` to disallow any errors that are thrown synchronously from the given function. Previously, throwing an error would cause the same behavior as returning a rejected Promise. Fixes: https://github.com/nodejs/node/issues/19646 PR-URL: https://github.com/nodejs/node/pull/19650 Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de>
74 lines
2.0 KiB
JavaScript
74 lines
2.0 KiB
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const { promisify } = require('util');
|
|
const wait = promisify(setTimeout);
|
|
|
|
/* eslint-disable prefer-common-expectserror, no-restricted-properties */
|
|
|
|
// Test assert.rejects() and assert.doesNotReject() by checking their
|
|
// expected output and by verifying that they do not work sync
|
|
|
|
common.crashOnUnhandledRejection();
|
|
|
|
(async () => {
|
|
await assert.rejects(
|
|
async () => assert.fail(),
|
|
common.expectsError({
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: 'Failed'
|
|
})
|
|
);
|
|
|
|
await assert.doesNotReject(() => {});
|
|
|
|
{
|
|
const promise = assert.doesNotReject(async () => {
|
|
await wait(1);
|
|
throw new Error();
|
|
});
|
|
await assert.rejects(
|
|
() => promise,
|
|
(err) => {
|
|
assert(err instanceof assert.AssertionError,
|
|
`${err.name} is not instance of AssertionError`);
|
|
assert.strictEqual(err.code, 'ERR_ASSERTION');
|
|
assert(/^Got unwanted rejection\.\n$/.test(err.message));
|
|
assert.strictEqual(err.operator, 'doesNotReject');
|
|
assert.ok(!err.stack.includes('at Function.doesNotReject'));
|
|
return true;
|
|
}
|
|
);
|
|
}
|
|
|
|
{
|
|
const promise = assert.rejects(() => {});
|
|
await assert.rejects(
|
|
() => promise,
|
|
(err) => {
|
|
assert(err instanceof assert.AssertionError,
|
|
`${err.name} is not instance of AssertionError`);
|
|
assert.strictEqual(err.code, 'ERR_ASSERTION');
|
|
assert(/^Missing expected rejection\.$/.test(err.message));
|
|
assert.strictEqual(err.operator, 'rejects');
|
|
assert.ok(!err.stack.includes('at Function.rejects'));
|
|
return true;
|
|
}
|
|
);
|
|
}
|
|
|
|
{
|
|
const THROWN_ERROR = new Error();
|
|
|
|
await assert.rejects(() => {
|
|
throw THROWN_ERROR;
|
|
}).then(common.mustNotCall())
|
|
.catch(
|
|
common.mustCall((err) => {
|
|
assert.strictEqual(err, THROWN_ERROR);
|
|
})
|
|
);
|
|
}
|
|
})().then(common.mustCall());
|