mirror of
https://github.com/nodejs/node.git
synced 2025-05-10 17:57:53 +00:00
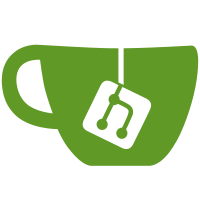
This adds missing async_hooks destroy calls for sockets (in _http_agent.js) and HTTP parsers. We need to emit a destroy in AsyncWrap#AsyncReset before assigning a new async_id when the instance has already been in use and is being recycled, because in that case, we have already emitted an init for the "old" async_id. This also removes a duplicated init call for HTTP parser: Each time a new parser was created, AsyncReset was being called via the C++ Parser class constructor (super constructor AsyncWrap) and also via Parser::Reinitialize. PR-URL: https://github.com/nodejs/node/pull/23272 Fixes: https://github.com/nodejs/node/issues/19859 Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Anna Henningsen <anna@addaleax.net> Reviewed-By: James M Snell <jasnell@gmail.com>
31 lines
955 B
JavaScript
31 lines
955 B
JavaScript
'use strict';
|
|
|
|
// Flags: --expose-internals
|
|
|
|
require('../common');
|
|
const assert = require('assert');
|
|
const { FreeList } = require('internal/freelist');
|
|
|
|
assert.strictEqual(typeof FreeList, 'function');
|
|
|
|
const flist1 = new FreeList('flist1', 3, Object);
|
|
|
|
// Allocating when empty, should not change the list size
|
|
const result = flist1.alloc();
|
|
assert.strictEqual(typeof result, 'object');
|
|
assert.strictEqual(flist1.list.length, 0);
|
|
|
|
// Exhaust the free list
|
|
assert(flist1.free({ id: 'test1' }));
|
|
assert(flist1.free({ id: 'test2' }));
|
|
assert(flist1.free({ id: 'test3' }));
|
|
|
|
// Now it should not return 'true', as max length is exceeded
|
|
assert.strictEqual(flist1.free({ id: 'test4' }), false);
|
|
assert.strictEqual(flist1.free({ id: 'test5' }), false);
|
|
|
|
// At this point 'alloc' should just return the stored values
|
|
assert.strictEqual(flist1.alloc().id, 'test3');
|
|
assert.strictEqual(flist1.alloc().id, 'test2');
|
|
assert.strictEqual(flist1.alloc().id, 'test1');
|