mirror of
https://github.com/nodejs/node.git
synced 2025-05-07 11:44:51 +00:00
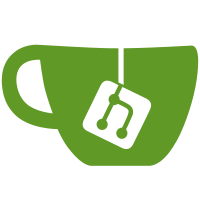
* Update the user timing implementation to conform to User Timing Level 3. * Reimplement user timing and timerify with pure JavaScript implementations * Simplify the C++ implementation for gc and http2 perf * Runtime deprecate additional perf entry properties in favor of the standard detail argument * Disable the `buffered` option on PerformanceObserver, all entries are queued and dispatched on setImmediate. Only entries with active observers are buffered. * This does remove the user timing and timerify trace events. Because the trace_events are still considered experimental, those can be removed without a deprecation cycle. They are removed to improve performance and reduce complexity. Old: `perf_hooks/usertiming.js n=100000: 92,378.01249733355` New: perf_hooks/usertiming.js n=100000: 270,393.5280638482` PR-URL: https://github.com/nodejs/node/pull/37136 Refs: https://github.com/nodejs/diagnostics/issues/464 Reviewed-By: Antoine du Hamel <duhamelantoine1995@gmail.com> Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com>
34 lines
875 B
JavaScript
34 lines
875 B
JavaScript
'use strict';
|
|
|
|
const nodeTiming = require('internal/perf/nodetiming');
|
|
|
|
const { now } = require('internal/perf/perf');
|
|
|
|
function eventLoopUtilization(util1, util2) {
|
|
const ls = nodeTiming.loopStart;
|
|
|
|
if (ls <= 0) {
|
|
return { idle: 0, active: 0, utilization: 0 };
|
|
}
|
|
|
|
if (util2) {
|
|
const idle = util1.idle - util2.idle;
|
|
const active = util1.active - util2.active;
|
|
return { idle, active, utilization: active / (idle + active) };
|
|
}
|
|
|
|
const idle = nodeTiming.idleTime;
|
|
const active = now() - ls - idle;
|
|
|
|
if (!util1) {
|
|
return { idle, active, utilization: active / (idle + active) };
|
|
}
|
|
|
|
const idle_delta = idle - util1.idle;
|
|
const active_delta = active - util1.active;
|
|
const utilization = active_delta / (idle_delta + active_delta);
|
|
return { idle: idle_delta, active: active_delta, utilization };
|
|
}
|
|
|
|
module.exports = eventLoopUtilization;
|