mirror of
https://github.com/nodejs/node.git
synced 2025-05-21 17:44:15 +00:00
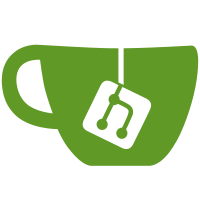
PR-URL: https://github.com/nodejs/node/pull/35908 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de> Reviewed-By: Myles Borins <myles.borins@gmail.com> Reviewed-By: Ruy Adorno <ruyadorno@github.com> Reviewed-By: Guy Bedford <guybedford@gmail.com>
65 lines
1.7 KiB
JavaScript
65 lines
1.7 KiB
JavaScript
// module to set the appropriate log settings based on configs
|
|
// returns a boolean to say whether we should enable color on
|
|
// stdout or not.
|
|
//
|
|
// Also (and this is a really inexcusable kludge), we patch the
|
|
// log.warn() method so that when we see a peerDep override
|
|
// explanation from Arborist, we can replace the object with a
|
|
// highly abbreviated explanation of what's being overridden.
|
|
const log = require('npmlog')
|
|
const { explain } = require('./explain-eresolve.js')
|
|
|
|
module.exports = (config) => {
|
|
const color = config.get('color')
|
|
|
|
const { warn } = log
|
|
|
|
log.warn = (heading, ...args) => {
|
|
if (heading === 'ERESOLVE' && args[1] && typeof args[1] === 'object') {
|
|
warn(heading, args[0])
|
|
return warn('', explain(args[1]))
|
|
}
|
|
return warn(heading, ...args)
|
|
}
|
|
|
|
if (config.get('timing') && config.get('loglevel') === 'notice')
|
|
log.level = 'timing'
|
|
else
|
|
log.level = config.get('loglevel')
|
|
|
|
log.heading = config.get('heading') || 'npm'
|
|
|
|
const stdoutTTY = process.stdout.isTTY
|
|
const stderrTTY = process.stderr.isTTY
|
|
const dumbTerm = process.env.TERM === 'dumb'
|
|
const stderrNotDumb = stderrTTY && !dumbTerm
|
|
|
|
const enableColorStderr = color === 'always' ? true
|
|
: color === false ? false
|
|
: stderrTTY
|
|
|
|
const enableColorStdout = color === 'always' ? true
|
|
: color === false ? false
|
|
: stdoutTTY
|
|
|
|
if (enableColorStderr)
|
|
log.enableColor()
|
|
else
|
|
log.disableColor()
|
|
|
|
if (config.get('unicode'))
|
|
log.enableUnicode()
|
|
else
|
|
log.disableUnicode()
|
|
|
|
// if it's more than error, don't show progress
|
|
const quiet = log.levels[log.level] > log.levels.error
|
|
|
|
if (config.get('progress') && stderrNotDumb && !quiet)
|
|
log.enableProgress()
|
|
else
|
|
log.disableProgress()
|
|
|
|
return enableColorStdout
|
|
}
|