mirror of
https://github.com/nodejs/node.git
synced 2025-05-06 21:35:34 +00:00
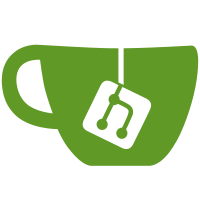
This commit renames all JavaScript source files in lib to lower snake_case. PR-URL: https://github.com/nodejs/node/pull/19556 Reviewed-By: Anna Henningsen <anna@addaleax.net> Reviewed-By: Trivikram Kamat <trivikr.dev@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Tiancheng "Timothy" Gu <timothygu99@gmail.com>
34 lines
964 B
JavaScript
34 lines
964 B
JavaScript
'use strict';
|
|
|
|
const ModuleJob = require('internal/modules/esm/module_job');
|
|
const { SafeMap } = require('internal/safe_globals');
|
|
const debug = require('util').debuglog('esm');
|
|
const { ERR_INVALID_ARG_TYPE } = require('internal/errors').codes;
|
|
|
|
// Tracks the state of the loader-level module cache
|
|
class ModuleMap extends SafeMap {
|
|
get(url) {
|
|
if (typeof url !== 'string') {
|
|
throw new ERR_INVALID_ARG_TYPE('url', 'string', url);
|
|
}
|
|
return super.get(url);
|
|
}
|
|
set(url, job) {
|
|
if (typeof url !== 'string') {
|
|
throw new ERR_INVALID_ARG_TYPE('url', 'string', url);
|
|
}
|
|
if (job instanceof ModuleJob !== true) {
|
|
throw new ERR_INVALID_ARG_TYPE('job', 'ModuleJob', job);
|
|
}
|
|
debug(`Storing ${url} in ModuleMap`);
|
|
return super.set(url, job);
|
|
}
|
|
has(url) {
|
|
if (typeof url !== 'string') {
|
|
throw new ERR_INVALID_ARG_TYPE('url', 'string', url);
|
|
}
|
|
return super.has(url);
|
|
}
|
|
}
|
|
module.exports = ModuleMap;
|