mirror of
https://github.com/nodejs/node.git
synced 2025-05-07 13:47:16 +00:00
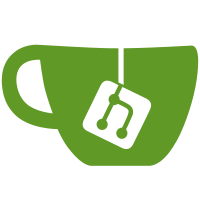
Evidence has shown that use of primordials have sometimes an impact of performance. This commit reverts the changes who are most likely to be responsible for performance regression in the HTTP response path. PR-URL: https://github.com/nodejs/node/pull/38248 Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com> Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com> Reviewed-By: Rich Trott <rtrott@gmail.com>
64 lines
1.5 KiB
JavaScript
64 lines
1.5 KiB
JavaScript
// LazyTransform is a special type of Transform stream that is lazily loaded.
|
|
// This is used for performance with bi-API-ship: when two APIs are available
|
|
// for the stream, one conventional and one non-conventional.
|
|
'use strict';
|
|
|
|
const {
|
|
ObjectDefineProperties,
|
|
ObjectDefineProperty,
|
|
ObjectSetPrototypeOf,
|
|
} = primordials;
|
|
|
|
const stream = require('stream');
|
|
|
|
const {
|
|
getDefaultEncoding
|
|
} = require('internal/crypto/util');
|
|
|
|
module.exports = LazyTransform;
|
|
|
|
function LazyTransform(options) {
|
|
this._options = options;
|
|
}
|
|
ObjectSetPrototypeOf(LazyTransform.prototype, stream.Transform.prototype);
|
|
ObjectSetPrototypeOf(LazyTransform, stream.Transform);
|
|
|
|
function makeGetter(name) {
|
|
return function() {
|
|
stream.Transform.call(this, this._options);
|
|
this._writableState.decodeStrings = false;
|
|
|
|
if (!this._options || !this._options.defaultEncoding) {
|
|
this._writableState.defaultEncoding = getDefaultEncoding();
|
|
}
|
|
|
|
return this[name];
|
|
};
|
|
}
|
|
|
|
function makeSetter(name) {
|
|
return function(val) {
|
|
ObjectDefineProperty(this, name, {
|
|
value: val,
|
|
enumerable: true,
|
|
configurable: true,
|
|
writable: true
|
|
});
|
|
};
|
|
}
|
|
|
|
ObjectDefineProperties(LazyTransform.prototype, {
|
|
_readableState: {
|
|
get: makeGetter('_readableState'),
|
|
set: makeSetter('_readableState'),
|
|
configurable: true,
|
|
enumerable: true
|
|
},
|
|
_writableState: {
|
|
get: makeGetter('_writableState'),
|
|
set: makeSetter('_writableState'),
|
|
configurable: true,
|
|
enumerable: true
|
|
}
|
|
});
|