mirror of
https://github.com/nodejs/node.git
synced 2025-05-02 17:01:08 +00:00
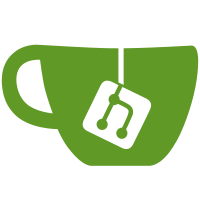
This commit adds special handling of Error instances when passed as the message argument to assert functions. With this commit, if an Error is passed as the message, then that Error is thrown instead of an AssertionError. PR-URL: https://github.com/nodejs/node/pull/15304 Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de>
94 lines
2.0 KiB
JavaScript
94 lines
2.0 KiB
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
|
|
// No args
|
|
assert.throws(
|
|
() => { assert.fail(); },
|
|
common.expectsError({
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: 'Failed',
|
|
operator: undefined,
|
|
actual: undefined,
|
|
expected: undefined
|
|
})
|
|
);
|
|
|
|
// One arg = message
|
|
common.expectsError(() => {
|
|
assert.fail('custom message');
|
|
}, {
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: 'custom message',
|
|
operator: undefined,
|
|
actual: undefined,
|
|
expected: undefined
|
|
});
|
|
|
|
// One arg = Error
|
|
common.expectsError(() => {
|
|
assert.fail(new TypeError('custom message'));
|
|
}, {
|
|
type: TypeError,
|
|
message: 'custom message',
|
|
operator: undefined,
|
|
actual: undefined,
|
|
expected: undefined
|
|
});
|
|
|
|
// Two args only, operator defaults to '!='
|
|
common.expectsError(() => {
|
|
assert.fail('first', 'second');
|
|
}, {
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: '\'first\' != \'second\'',
|
|
operator: '!=',
|
|
actual: 'first',
|
|
expected: 'second'
|
|
});
|
|
|
|
// Three args
|
|
common.expectsError(() => {
|
|
assert.fail('ignored', 'ignored', 'another custom message');
|
|
}, {
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: 'another custom message',
|
|
operator: undefined,
|
|
actual: 'ignored',
|
|
expected: 'ignored'
|
|
});
|
|
|
|
// Three args with custom Error
|
|
common.expectsError(() => {
|
|
assert.fail(typeof 1, 'object', new TypeError('another custom message'));
|
|
}, {
|
|
type: TypeError,
|
|
message: 'another custom message',
|
|
operator: undefined,
|
|
actual: 'number',
|
|
expected: 'object'
|
|
});
|
|
|
|
// No third arg (but a fourth arg)
|
|
common.expectsError(() => {
|
|
assert.fail('first', 'second', undefined, 'operator');
|
|
}, {
|
|
code: 'ERR_ASSERTION',
|
|
type: assert.AssertionError,
|
|
message: '\'first\' operator \'second\'',
|
|
operator: 'operator',
|
|
actual: 'first',
|
|
expected: 'second'
|
|
});
|
|
|
|
// The stackFrameFunction should exclude the foo frame
|
|
assert.throws(
|
|
function foo() { assert.fail('first', 'second', 'message', '!==', foo); },
|
|
(err) => !/^\s*at\sfoo\b/m.test(err.stack)
|
|
);
|