mirror of
https://github.com/nodejs/node.git
synced 2025-05-02 13:11:36 +00:00
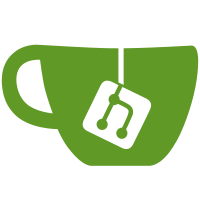
This fixes validating `EventListener` given to `add/removeEventListener` to improve the Web API compatibility. According to the WPT test failure with the current validation, `addEventListener` should not require `handleEvent` to be defined on an `EventListener`". IIUC, the same can be applied to `removeEventListener` also. Signed-off-by: Daeyeon Jeong daeyeon.dev@gmail.com PR-URL: https://github.com/nodejs/node/pull/43491 Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com> Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
120 lines
3.2 KiB
JavaScript
120 lines
3.2 KiB
JavaScript
'use strict';
|
|
|
|
require('../common');
|
|
const { test, assert_equals, assert_unreached } =
|
|
require('../common/wpt').harness;
|
|
|
|
// Manually ported from: https://github.com/web-platform-tests/wpt/blob/6cef1d2087d6a07d7cc6cee8cf207eec92e27c5f/dom/events/EventTarget-this-of-listener.html
|
|
|
|
// Mock document
|
|
const document = {
|
|
createElement: () => new EventTarget(),
|
|
createTextNode: () => new EventTarget(),
|
|
createDocumentFragment: () => new EventTarget(),
|
|
createComment: () => new EventTarget(),
|
|
createProcessingInstruction: () => new EventTarget(),
|
|
};
|
|
|
|
test(() => {
|
|
const nodes = [
|
|
document.createElement('p'),
|
|
document.createTextNode('some text'),
|
|
document.createDocumentFragment(),
|
|
document.createComment('a comment'),
|
|
document.createProcessingInstruction('target', 'data'),
|
|
];
|
|
|
|
let callCount = 0;
|
|
for (const node of nodes) {
|
|
node.addEventListener('someevent', function() {
|
|
++callCount;
|
|
assert_equals(this, node);
|
|
});
|
|
|
|
node.dispatchEvent(new Event('someevent'));
|
|
}
|
|
|
|
assert_equals(callCount, nodes.length);
|
|
}, 'the this value inside the event listener callback should be the node');
|
|
|
|
test(() => {
|
|
const nodes = [
|
|
document.createElement('p'),
|
|
document.createTextNode('some text'),
|
|
document.createDocumentFragment(),
|
|
document.createComment('a comment'),
|
|
document.createProcessingInstruction('target', 'data'),
|
|
];
|
|
|
|
let callCount = 0;
|
|
for (const node of nodes) {
|
|
const handler = {};
|
|
|
|
node.addEventListener('someevent', handler);
|
|
handler.handleEvent = function() {
|
|
++callCount;
|
|
assert_equals(this, handler);
|
|
};
|
|
|
|
node.dispatchEvent(new Event('someevent'));
|
|
}
|
|
|
|
assert_equals(callCount, nodes.length);
|
|
}, 'addEventListener should not require handleEvent to be defined on object listeners');
|
|
|
|
test(() => {
|
|
const nodes = [
|
|
document.createElement('p'),
|
|
document.createTextNode('some text'),
|
|
document.createDocumentFragment(),
|
|
document.createComment('a comment'),
|
|
document.createProcessingInstruction('target', 'data'),
|
|
];
|
|
|
|
let callCount = 0;
|
|
for (const node of nodes) {
|
|
function handler() {
|
|
++callCount;
|
|
assert_equals(this, node);
|
|
}
|
|
|
|
handler.handleEvent = () => {
|
|
assert_unreached('should not call the handleEvent method on a function');
|
|
};
|
|
|
|
node.addEventListener('someevent', handler);
|
|
|
|
node.dispatchEvent(new Event('someevent'));
|
|
}
|
|
|
|
assert_equals(callCount, nodes.length);
|
|
}, 'handleEvent properties added to a function before addEventListener are not reached');
|
|
|
|
test(() => {
|
|
const nodes = [
|
|
document.createElement('p'),
|
|
document.createTextNode('some text'),
|
|
document.createDocumentFragment(),
|
|
document.createComment('a comment'),
|
|
document.createProcessingInstruction('target', 'data'),
|
|
];
|
|
|
|
let callCount = 0;
|
|
for (const node of nodes) {
|
|
function handler() {
|
|
++callCount;
|
|
assert_equals(this, node);
|
|
}
|
|
|
|
node.addEventListener('someevent', handler);
|
|
|
|
handler.handleEvent = () => {
|
|
assert_unreached('should not call the handleEvent method on a function');
|
|
};
|
|
|
|
node.dispatchEvent(new Event('someevent'));
|
|
}
|
|
|
|
assert_equals(callCount, nodes.length);
|
|
}, 'handleEvent properties added to a function after addEventListener are not reached');
|