mirror of
https://github.com/nodejs/node.git
synced 2025-05-15 19:07:23 +00:00
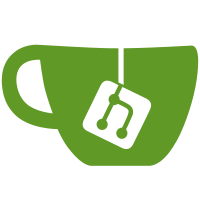
doc: update ESM hook examples esm: fix unsafe primordial doc: fix ESM example linting esm: allow source of type ArrayBuffer doc: update ESM hook changelog to include resolve format esm: allow all ArrayBuffers and TypedArrays for load hook source doc: tidy code & API docs doc: convert ESM source table header from Title Case to Sentence case doc: add detailed explanation for getPackageType esm: add caveat that ESMLoader::import() must NOT be renamed esm: tidy code declaration of getFormat protocolHandlers doc: correct ESM doc link (bad conflict resolution) doc: update ESM hook limitation for CJS esm: tweak preload description doc: update ESM getPackageType() example explanation PR-URL: https://github.com/nodejs/node/pull/37468 Reviewed-By: Antoine du Hamel <duhamelantoine1995@gmail.com> Reviewed-By: Guy Bedford <guybedford@gmail.com> Reviewed-By: Bradley Farias <bradley.meck@gmail.com> Reviewed-By: Geoffrey Booth <webmaster@geoffreybooth.com>
46 lines
1.3 KiB
JavaScript
46 lines
1.3 KiB
JavaScript
'use strict';
|
|
|
|
const {
|
|
RegExpPrototypeExec,
|
|
decodeURIComponent,
|
|
} = primordials;
|
|
const { getOptionValue } = require('internal/options');
|
|
// Do not eagerly grab .manifest, it may be in TDZ
|
|
const policy = getOptionValue('--experimental-policy') ?
|
|
require('internal/process/policy') :
|
|
null;
|
|
|
|
const { Buffer } = require('buffer');
|
|
|
|
const fs = require('internal/fs/promises').exports;
|
|
const { URL } = require('internal/url');
|
|
const {
|
|
ERR_INVALID_URL,
|
|
ERR_INVALID_URL_SCHEME,
|
|
} = require('internal/errors').codes;
|
|
const readFileAsync = fs.readFile;
|
|
|
|
const DATA_URL_PATTERN = /^[^/]+\/[^,;]+(?:[^,]*?)(;base64)?,([\s\S]*)$/;
|
|
|
|
async function defaultGetSource(url, { format } = {}, defaultGetSource) {
|
|
const parsed = new URL(url);
|
|
let source;
|
|
if (parsed.protocol === 'file:') {
|
|
source = await readFileAsync(parsed);
|
|
} else if (parsed.protocol === 'data:') {
|
|
const match = RegExpPrototypeExec(DATA_URL_PATTERN, parsed.pathname);
|
|
if (!match) {
|
|
throw new ERR_INVALID_URL(url);
|
|
}
|
|
const { 1: base64, 2: body } = match;
|
|
source = Buffer.from(decodeURIComponent(body), base64 ? 'base64' : 'utf8');
|
|
} else {
|
|
throw new ERR_INVALID_URL_SCHEME(['file', 'data']);
|
|
}
|
|
if (policy?.manifest) {
|
|
policy.manifest.assertIntegrity(parsed, source);
|
|
}
|
|
return source;
|
|
}
|
|
exports.defaultGetSource = defaultGetSource;
|