mirror of
https://github.com/nodejs/node.git
synced 2025-05-01 17:03:34 +00:00
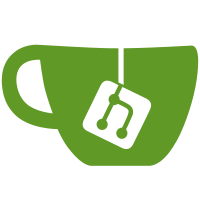
We were previously reading from the wrong offset, namely the one into the final results array, not the one for the AAAA results itself, which could have lead to reading uninitialized or out-of-bounds data. Also, adjust the test accordingly; TTL values are not modified by c-ares, but are only exposed for a subset of all DNS record types. PR-URL: https://github.com/nodejs/node/pull/25187 Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Khaidi Chu <i@2333.moe> Reviewed-By: Ben Noordhuis <info@bnoordhuis.nl> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de>
66 lines
1.8 KiB
JavaScript
66 lines
1.8 KiB
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const dnstools = require('../common/dns');
|
|
const dns = require('dns');
|
|
const assert = require('assert');
|
|
const dgram = require('dgram');
|
|
const dnsPromises = dns.promises;
|
|
|
|
const answers = [
|
|
{ type: 'A', address: '1.2.3.4', ttl: 123 },
|
|
{ type: 'AAAA', address: '::42', ttl: 123 },
|
|
{ type: 'MX', priority: 42, exchange: 'foobar.com', ttl: 124 },
|
|
{ type: 'NS', value: 'foobar.org', ttl: 457 },
|
|
{ type: 'TXT', entries: [ 'v=spf1 ~all', 'xyz' ] },
|
|
{ type: 'PTR', value: 'baz.org', ttl: 987 },
|
|
{
|
|
type: 'SOA',
|
|
nsname: 'ns1.example.com',
|
|
hostmaster: 'admin.example.com',
|
|
serial: 156696742,
|
|
refresh: 900,
|
|
retry: 900,
|
|
expire: 1800,
|
|
minttl: 60
|
|
},
|
|
];
|
|
|
|
const server = dgram.createSocket('udp4');
|
|
|
|
server.on('message', common.mustCall((msg, { address, port }) => {
|
|
const parsed = dnstools.parseDNSPacket(msg);
|
|
const domain = parsed.questions[0].domain;
|
|
assert.strictEqual(domain, 'example.org');
|
|
|
|
server.send(dnstools.writeDNSPacket({
|
|
id: parsed.id,
|
|
questions: parsed.questions,
|
|
answers: answers.map((answer) => Object.assign({ domain }, answer)),
|
|
}), port, address);
|
|
}, 2));
|
|
|
|
server.bind(0, common.mustCall(async () => {
|
|
const address = server.address();
|
|
dns.setServers([`127.0.0.1:${address.port}`]);
|
|
|
|
validateResults(await dnsPromises.resolveAny('example.org'));
|
|
|
|
dns.resolveAny('example.org', common.mustCall((err, res) => {
|
|
assert.ifError(err);
|
|
validateResults(res);
|
|
server.close();
|
|
}));
|
|
}));
|
|
|
|
function validateResults(res) {
|
|
// TTL values are only provided for A and AAAA entries.
|
|
assert.deepStrictEqual(res.map(maybeRedactTTL), answers.map(maybeRedactTTL));
|
|
}
|
|
|
|
function maybeRedactTTL(r) {
|
|
const ret = { ...r };
|
|
if (!['A', 'AAAA'].includes(r.type))
|
|
delete ret.ttl;
|
|
return ret;
|
|
}
|