mirror of
https://github.com/nodejs/node.git
synced 2025-05-19 02:54:19 +00:00
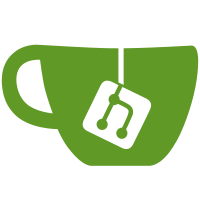
PR-URL: https://github.com/nodejs/io.js/pull/2072 Reviewed-By: Yosuke Furukawa <yosuke.furukawa@gmail.com> Reviewed-by: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: Alex Kocharin <alex@kocharin.ru>
62 lines
1.7 KiB
JavaScript
62 lines
1.7 KiB
JavaScript
/**
|
|
* @fileoverview A rule to set the maximum number of statements in a function.
|
|
* @author Ian Christian Myers
|
|
* @copyright 2013 Ian Christian Myers. All rights reserved.
|
|
*/
|
|
|
|
"use strict";
|
|
|
|
//------------------------------------------------------------------------------
|
|
// Rule Definition
|
|
//------------------------------------------------------------------------------
|
|
|
|
module.exports = function(context) {
|
|
|
|
//--------------------------------------------------------------------------
|
|
// Helpers
|
|
//--------------------------------------------------------------------------
|
|
|
|
var functionStack = [],
|
|
maxStatements = context.options[0] || 10;
|
|
|
|
function startFunction() {
|
|
functionStack.push(0);
|
|
}
|
|
|
|
function endFunction(node) {
|
|
var count = functionStack.pop();
|
|
|
|
if (count > maxStatements) {
|
|
context.report(node, "This function has too many statements ({{count}}). Maximum allowed is {{max}}.",
|
|
{ count: count, max: maxStatements });
|
|
}
|
|
}
|
|
|
|
function countStatements(node) {
|
|
functionStack[functionStack.length - 1] += node.body.length;
|
|
}
|
|
|
|
//--------------------------------------------------------------------------
|
|
// Public API
|
|
//--------------------------------------------------------------------------
|
|
|
|
return {
|
|
"FunctionDeclaration": startFunction,
|
|
"FunctionExpression": startFunction,
|
|
"ArrowFunctionExpression": startFunction,
|
|
|
|
"BlockStatement": countStatements,
|
|
|
|
"FunctionDeclaration:exit": endFunction,
|
|
"FunctionExpression:exit": endFunction,
|
|
"ArrowFunctionExpression:exit": endFunction
|
|
};
|
|
|
|
};
|
|
|
|
module.exports.schema = [
|
|
{
|
|
"type": "integer"
|
|
}
|
|
];
|