mirror of
https://github.com/nodejs/node.git
synced 2025-05-11 09:57:00 +00:00
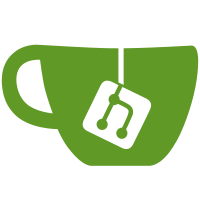
- Remove `NativeModule._source` - the compilation is now entirely done in C++ and `process.binding('natives')` is implemented directly in the binding loader so there is no need to store additional source code strings. - Instead of using an object as `NativeModule._cached` and insert into it after compilation of each native module, simply prebuild a JS map filled with all the native modules and infer the state of compilation through `mod.loading`/`mod.loaded`. - Rename `NativeModule.nonInternalExists` to `NativeModule.canBeRequiredByUsers` and precompute that property for all the native modules during bootstrap instead of branching in every require call during runtime. This also fixes the bug where `worker_threads` can be made available with `--expose-internals`. - Rename `NativeModule.requireForDeps` to `NativeModule.requireWithFallbackInDeps`. - Add a test to make sure we do not accidentally leak any module to the global namespace. PR-URL: https://github.com/nodejs/node/pull/25352 Reviewed-By: Anna Henningsen <anna@addaleax.net>
82 lines
2.0 KiB
JavaScript
82 lines
2.0 KiB
JavaScript
'use strict';
|
|
|
|
// This is only exposed for internal build steps and testing purposes.
|
|
// We create new copies of the source and the code cache
|
|
// so the resources eventually used to compile builtin modules
|
|
// cannot be tampered with even with --expose-internals.
|
|
|
|
const { NativeModule } = require('internal/bootstrap/loaders');
|
|
const {
|
|
getCodeCache, compileFunction
|
|
} = internalBinding('native_module');
|
|
const { hasTracing, hasInspector } = process.binding('config');
|
|
|
|
// Modules with source code compiled in js2c that
|
|
// cannot be compiled with the code cache.
|
|
const cannotUseCache = [
|
|
'sys', // Deprecated.
|
|
'internal/v8_prof_polyfill',
|
|
'internal/v8_prof_processor',
|
|
|
|
'internal/per_context',
|
|
|
|
'internal/test/binding',
|
|
// TODO(joyeecheung): update the C++ side so that
|
|
// the code cache is also used when compiling these two files.
|
|
'internal/bootstrap/loaders',
|
|
'internal/bootstrap/node'
|
|
];
|
|
|
|
// Skip modules that cannot be required when they are not
|
|
// built into the binary.
|
|
if (!hasInspector) {
|
|
cannotUseCache.push(
|
|
'inspector',
|
|
'internal/util/inspector',
|
|
);
|
|
}
|
|
if (!hasTracing) {
|
|
cannotUseCache.push('trace_events');
|
|
}
|
|
if (!process.versions.openssl) {
|
|
cannotUseCache.push(
|
|
'crypto',
|
|
'https',
|
|
'http2',
|
|
'tls',
|
|
'_tls_common',
|
|
'_tls_wrap',
|
|
'internal/crypto/certificate',
|
|
'internal/crypto/cipher',
|
|
'internal/crypto/diffiehellman',
|
|
'internal/crypto/hash',
|
|
'internal/crypto/keygen',
|
|
'internal/crypto/keys',
|
|
'internal/crypto/pbkdf2',
|
|
'internal/crypto/random',
|
|
'internal/crypto/scrypt',
|
|
'internal/crypto/sig',
|
|
'internal/crypto/util',
|
|
'internal/http2/core',
|
|
'internal/http2/compat',
|
|
'internal/streams/lazy_transform',
|
|
);
|
|
}
|
|
|
|
const cachableBuiltins = [];
|
|
for (const id of NativeModule.map.keys()) {
|
|
if (id.startsWith('internal/deps')) {
|
|
cannotUseCache.push(id);
|
|
}
|
|
if (!cannotUseCache.includes(id)) {
|
|
cachableBuiltins.push(id);
|
|
}
|
|
}
|
|
|
|
module.exports = {
|
|
cachableBuiltins,
|
|
getCodeCache,
|
|
compileFunction,
|
|
cannotUseCache
|
|
};
|