mirror of
https://github.com/nodejs/node.git
synced 2025-05-04 23:42:17 +00:00
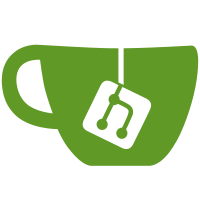
Streams were recently updated to emit their own close event. The Http2Stream was an exception because it included the close argument with the close event. Refactor that to use the built in close. PR-URL: https://github.com/nodejs/node/pull/19451 Reviewed-By: Matteo Collina <matteo.collina@gmail.com>
70 lines
1.7 KiB
JavaScript
70 lines
1.7 KiB
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
const assert = require('assert');
|
|
const h2 = require('http2');
|
|
|
|
const server = h2.createServer();
|
|
server.on('stream', (stream) => {
|
|
stream.on('close', common.mustCall());
|
|
stream.respond();
|
|
stream.end('ok');
|
|
});
|
|
|
|
server.listen(0, common.mustCall(() => {
|
|
const client = h2.connect(`http://localhost:${server.address().port}`);
|
|
const req = client.request();
|
|
const closeCode = 1;
|
|
|
|
common.expectsError(
|
|
() => req.close(2 ** 32),
|
|
{
|
|
type: RangeError,
|
|
code: 'ERR_OUT_OF_RANGE',
|
|
message: 'The value of "code" is out of range.'
|
|
}
|
|
);
|
|
assert.strictEqual(req.closed, false);
|
|
|
|
[true, 1, {}, [], null, 'test'].forEach((notFunction) => {
|
|
common.expectsError(
|
|
() => req.close(closeCode, notFunction),
|
|
{
|
|
type: TypeError,
|
|
code: 'ERR_INVALID_CALLBACK',
|
|
message: 'Callback must be a function'
|
|
}
|
|
);
|
|
assert.strictEqual(req.closed, false);
|
|
});
|
|
|
|
req.close(closeCode, common.mustCall());
|
|
assert.strictEqual(req.closed, true);
|
|
|
|
// Make sure that destroy is called.
|
|
req._destroy = common.mustCall(req._destroy.bind(req));
|
|
|
|
// Second call doesn't do anything.
|
|
req.close(closeCode + 1);
|
|
|
|
req.on('close', common.mustCall(() => {
|
|
assert.strictEqual(req.destroyed, true);
|
|
assert.strictEqual(req.rstCode, closeCode);
|
|
server.close();
|
|
client.close();
|
|
}));
|
|
|
|
req.on('error', common.expectsError({
|
|
code: 'ERR_HTTP2_STREAM_ERROR',
|
|
type: Error,
|
|
message: 'Stream closed with error code NGHTTP2_PROTOCOL_ERROR'
|
|
}));
|
|
|
|
req.on('response', common.mustCall());
|
|
req.resume();
|
|
req.on('end', common.mustCall());
|
|
req.end();
|
|
}));
|