mirror of
https://github.com/nodejs/node.git
synced 2025-05-01 08:42:45 +00:00
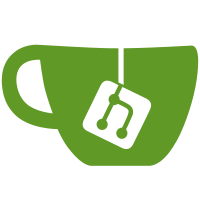
This change makes the error codes returned by v8.writeHeapSnapshot() consistent across all platforms by using the libuv APIs instead of fopen(), fwrite() and fclose(). This also starts reporting potential errors that might happen during the write operations. Signed-off-by: Darshan Sen <raisinten@gmail.com> PR-URL: https://github.com/nodejs/node/pull/42577 Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Joyee Cheung <joyeec9h3@gmail.com> Reviewed-By: Anna Henningsen <anna@addaleax.net>
61 lines
1.4 KiB
JavaScript
61 lines
1.4 KiB
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
|
|
if (!common.isMainThread)
|
|
common.skip('process.chdir is not available in Workers');
|
|
|
|
const { writeHeapSnapshot, getHeapSnapshot } = require('v8');
|
|
const assert = require('assert');
|
|
const fs = require('fs');
|
|
const tmpdir = require('../common/tmpdir');
|
|
|
|
tmpdir.refresh();
|
|
process.chdir(tmpdir.path);
|
|
|
|
{
|
|
writeHeapSnapshot('my.heapdump');
|
|
fs.accessSync('my.heapdump');
|
|
}
|
|
|
|
{
|
|
const heapdump = writeHeapSnapshot();
|
|
assert.strictEqual(typeof heapdump, 'string');
|
|
fs.accessSync(heapdump);
|
|
}
|
|
|
|
{
|
|
const directory = 'directory';
|
|
fs.mkdirSync(directory);
|
|
assert.throws(() => {
|
|
writeHeapSnapshot(directory);
|
|
}, (e) => {
|
|
assert.ok(e, 'writeHeapSnapshot should error');
|
|
assert.strictEqual(e.code, 'EISDIR');
|
|
assert.strictEqual(e.syscall, 'open');
|
|
return true;
|
|
});
|
|
}
|
|
|
|
[1, true, {}, [], null, Infinity, NaN].forEach((i) => {
|
|
assert.throws(() => writeHeapSnapshot(i), {
|
|
code: 'ERR_INVALID_ARG_TYPE',
|
|
name: 'TypeError',
|
|
message: 'The "path" argument must be of type string or an instance of ' +
|
|
'Buffer or URL.' +
|
|
common.invalidArgTypeHelper(i)
|
|
});
|
|
});
|
|
|
|
{
|
|
let data = '';
|
|
const snapshot = getHeapSnapshot();
|
|
snapshot.setEncoding('utf-8');
|
|
snapshot.on('data', common.mustCallAtLeast((chunk) => {
|
|
data += chunk.toString();
|
|
}));
|
|
snapshot.on('end', common.mustCall(() => {
|
|
JSON.parse(data);
|
|
}));
|
|
}
|