mirror of
https://github.com/nodejs/node.git
synced 2025-05-01 08:42:45 +00:00
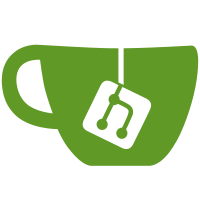
Having an experimental feature behind a flag makes change if we are expecting significant breaking changes to its API. Since the Worker API has been essentially stable since its initial introduction, and no noticeable doubt about possibly not keeping the feature around has been voiced, removing the flag and thereby reducing the barrier to experimentation, and consequently receiving feedback on the implementation, seems like a good idea. PR-URL: https://github.com/nodejs/node/pull/25361 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: Yuta Hiroto <hello@hiroppy.me> Reviewed-By: Shingo Inoue <leko.noor@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com> Reviewed-By: Tiancheng "Timothy" Gu <timothygu99@gmail.com> Reviewed-By: Tobias Nießen <tniessen@tnie.de> Reviewed-By: Masashi Hirano <shisama07@gmail.com> Reviewed-By: Weijia Wang <starkwang@126.com> Reviewed-By: Gireesh Punathil <gpunathi@in.ibm.com> Reviewed-By: Michael Dawson <michael_dawson@ca.ibm.com>
55 lines
2.0 KiB
JavaScript
55 lines
2.0 KiB
JavaScript
// Flags: --experimental-wasm-threads
|
|
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const { MessageChannel, Worker } = require('worker_threads');
|
|
|
|
// Test that SharedArrayBuffer instances created from WASM are transferrable
|
|
// through MessageChannels (without crashing).
|
|
|
|
const fixtures = require('../common/fixtures');
|
|
const wasmSource = fixtures.readSync('shared-memory.wasm');
|
|
const wasmModule = new WebAssembly.Module(wasmSource);
|
|
const instance = new WebAssembly.Instance(wasmModule);
|
|
|
|
const { buffer } = instance.exports.memory;
|
|
assert(buffer instanceof SharedArrayBuffer);
|
|
|
|
{
|
|
const { port1, port2 } = new MessageChannel();
|
|
port1.postMessage(buffer);
|
|
port2.once('message', common.mustCall((buffer2) => {
|
|
// Make sure serialized + deserialized buffer refer to the same memory.
|
|
const expected = 'Hello, world!';
|
|
const bytes = Buffer.from(buffer).write(expected);
|
|
const deserialized = Buffer.from(buffer2).toString('utf8', 0, bytes);
|
|
assert.deepStrictEqual(deserialized, expected);
|
|
}));
|
|
}
|
|
|
|
{
|
|
// Make sure we can free WASM memory originating from a thread that already
|
|
// stopped when we exit.
|
|
const worker = new Worker(`
|
|
const { parentPort } = require('worker_threads');
|
|
|
|
// Compile the same WASM module from its source bytes.
|
|
const wasmSource = new Uint8Array([${wasmSource.join(',')}]);
|
|
const wasmModule = new WebAssembly.Module(wasmSource);
|
|
const instance = new WebAssembly.Instance(wasmModule);
|
|
parentPort.postMessage(instance.exports.memory);
|
|
|
|
// Do the same thing, except we receive the WASM module via transfer.
|
|
parentPort.once('message', ({ wasmModule }) => {
|
|
const instance = new WebAssembly.Instance(wasmModule);
|
|
parentPort.postMessage(instance.exports.memory);
|
|
});
|
|
`, { eval: true });
|
|
worker.on('message', common.mustCall(({ buffer }) => {
|
|
assert(buffer instanceof SharedArrayBuffer);
|
|
worker.buf = buffer; // Basically just keep the reference to buffer alive.
|
|
}, 2));
|
|
worker.once('exit', common.mustCall());
|
|
worker.postMessage({ wasmModule });
|
|
}
|