mirror of
https://github.com/nodejs/node.git
synced 2025-04-30 23:56:58 +00:00
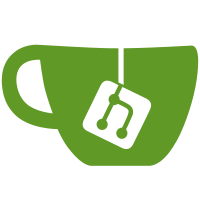
We need to emit dependency of ICU's toASCII in order to update the WPT fixtures. Since ICU and URL isn't the same implementation and they also follow different specifications. ICU's toASCII shouldn't have a dependency on WPT fixtures. Refs: https://github.com/nodejs/node/pull/33770#issuecomment-680853608 PR-URL: https://github.com/nodejs/node/pull/35077 Reviewed-By: Daijiro Wachi <daijiro.wachi@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com> Reviewed-By: Joyee Cheung <joyeec9h3@gmail.com>
58 lines
1.9 KiB
JavaScript
58 lines
1.9 KiB
JavaScript
'use strict';
|
|
// Flags: --expose-internals
|
|
const common = require('../common');
|
|
|
|
if (!common.hasIntl)
|
|
common.skip('missing Intl');
|
|
|
|
const { internalBinding } = require('internal/test/binding');
|
|
const icu = internalBinding('icu');
|
|
const assert = require('assert');
|
|
|
|
// Test hasConverter method
|
|
assert(icu.hasConverter('utf-8'),
|
|
'hasConverter should report converter exists for utf-8');
|
|
assert(!icu.hasConverter('x'),
|
|
'hasConverter should report converter does not exist for x');
|
|
|
|
const tests = require('../fixtures/url-idna.js');
|
|
const fixtures = require('../fixtures/icu-punycode-toascii.json');
|
|
|
|
{
|
|
for (const [i, { ascii, unicode }] of tests.entries()) {
|
|
assert.strictEqual(ascii, icu.toASCII(unicode), `toASCII(${i + 1})`);
|
|
assert.strictEqual(unicode, icu.toUnicode(ascii), `toUnicode(${i + 1})`);
|
|
assert.strictEqual(ascii, icu.toASCII(icu.toUnicode(ascii)),
|
|
`toASCII(toUnicode(${i + 1}))`);
|
|
assert.strictEqual(unicode, icu.toUnicode(icu.toASCII(unicode)),
|
|
`toUnicode(toASCII(${i + 1}))`);
|
|
}
|
|
}
|
|
|
|
{
|
|
for (const [i, test] of fixtures.entries()) {
|
|
if (typeof test === 'string')
|
|
continue; // skip comments
|
|
const { comment, input, output } = test;
|
|
let caseComment = `case ${i + 1}`;
|
|
if (comment)
|
|
caseComment += ` (${comment})`;
|
|
if (output === null) {
|
|
assert.throws(
|
|
() => icu.toASCII(input),
|
|
{
|
|
code: 'ERR_INVALID_ARG_VALUE',
|
|
name: 'TypeError',
|
|
message: 'Cannot convert name to ASCII'
|
|
}
|
|
);
|
|
icu.toASCII(input, true); // Should not throw.
|
|
} else {
|
|
assert.strictEqual(icu.toASCII(input), output, `ToASCII ${caseComment}`);
|
|
assert.strictEqual(icu.toASCII(input, true), output,
|
|
`ToASCII ${caseComment} in lenient mode`);
|
|
}
|
|
icu.toUnicode(input); // Should not throw.
|
|
}
|
|
}
|