mirror of
https://github.com/nodejs/node.git
synced 2025-05-01 08:42:45 +00:00
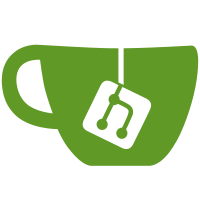
If we are waiting for the ability to send more output, we should not process more input. This commit a) makes us send output earlier, during processing of input, if we accumulate a lot and b) allows interrupting the call into nghttp2 that processes input data and resuming it at a later time, if we do find ourselves in a position where we are waiting to be able to send more output. This is part of mitigating CVE-2019-9511/CVE-2019-9517. PR-URL: https://github.com/nodejs/node/pull/29122 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
40 lines
996 B
JavaScript
40 lines
996 B
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
|
|
const fixtures = require('../common/fixtures');
|
|
const assert = require('assert');
|
|
const http2 = require('http2');
|
|
|
|
const content = fixtures.readSync('person-large.jpg');
|
|
|
|
const server = http2.createServer({
|
|
maxSessionMemory: 1000
|
|
});
|
|
server.on('stream', (stream, headers) => {
|
|
stream.respond({
|
|
'content-type': 'image/jpeg',
|
|
':status': 200
|
|
});
|
|
stream.end(content);
|
|
});
|
|
server.unref();
|
|
|
|
server.listen(0, common.mustCall(() => {
|
|
const client = http2.connect(`http://localhost:${server.address().port}/`);
|
|
|
|
let finished = 0;
|
|
for (let i = 0; i < 100; i++) {
|
|
const req = client.request({ ':path': '/' }).end();
|
|
const chunks = [];
|
|
req.on('data', (chunk) => {
|
|
chunks.push(chunk);
|
|
});
|
|
req.on('end', common.mustCall(() => {
|
|
assert.deepStrictEqual(Buffer.concat(chunks), content);
|
|
if (++finished === 100) client.close();
|
|
}));
|
|
}
|
|
}));
|