mirror of
https://github.com/nodejs/node.git
synced 2025-05-06 09:02:40 +00:00
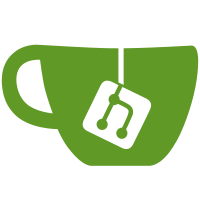
As per ecma-262 2015's #sec-%typedarray%-buffer-byteoffset-length, `offset` would be an integer, not a 32 bit unsigned integer. Also, `length` would be an integer with the maximum value of 2^53 - 1, not a 32 bit unsigned integer. This would be a problem because, if we create a buffer from an arraybuffer, from an offset which is greater than 2^32, it would be actually pointing to a different location in arraybuffer. For example, if we use 2^40 as offset, then the actual value used will be 0, because `byteOffset >>>= 0` will convert `byteOffset` to a 32 bit unsigned int, which is based on 2^32 modulo. PR-URL: https://github.com/nodejs/node/pull/9492 Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Trevor Norris <trev.norris@gmail.com>
22 lines
518 B
JavaScript
22 lines
518 B
JavaScript
'use strict';
|
|
|
|
require('../common');
|
|
const assert = require('assert');
|
|
|
|
function test(size, offset, length) {
|
|
const arrayBuffer = new ArrayBuffer(size);
|
|
|
|
const uint8Array = new Uint8Array(arrayBuffer, offset, length);
|
|
for (let i = 0; i < length; i += 1) {
|
|
uint8Array[i] = 1;
|
|
}
|
|
|
|
const buffer = Buffer.from(arrayBuffer, offset, length);
|
|
for (let i = 0; i < length; i += 1) {
|
|
assert.strictEqual(buffer[i], 1);
|
|
}
|
|
}
|
|
|
|
test(200, 50, 100);
|
|
test(8589934592 /* 1 << 40 */, 4294967296 /* 1 << 39 */, 1000);
|