mirror of
https://github.com/nodejs/node.git
synced 2025-05-09 07:27:32 +00:00
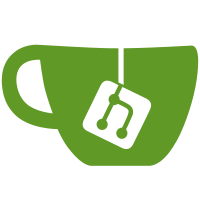
Handle edge case where stream pause is called between resume being called and actually evaluated. Other minor adjustments to avoid various edge cases around stream events. Add new tests that cover all changes. Fixes: https://github.com/nodejs/node/issues/15491 PR-URL: https://github.com/nodejs/node/pull/15503 Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
49 lines
1.3 KiB
JavaScript
49 lines
1.3 KiB
JavaScript
// Flags: --expose-http2
|
|
'use strict';
|
|
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
const assert = require('assert');
|
|
const http2 = require('http2');
|
|
const fs = require('fs');
|
|
const path = require('path');
|
|
|
|
// piping should work as expected with createWriteStream
|
|
|
|
const loc = path.join(common.fixturesDir, 'person.jpg');
|
|
const fn = path.join(common.tmpDir, 'http2pipe.jpg');
|
|
common.refreshTmpDir();
|
|
|
|
const server = http2.createServer();
|
|
|
|
server.on('request', common.mustCall((req, res) => {
|
|
const dest = req.pipe(fs.createWriteStream(fn));
|
|
dest.on('finish', common.mustCall(() => {
|
|
assert.deepStrictEqual(fs.readFileSync(loc), fs.readFileSync(fn));
|
|
fs.unlinkSync(fn);
|
|
res.end();
|
|
}));
|
|
}));
|
|
|
|
server.listen(0, common.mustCall(() => {
|
|
const port = server.address().port;
|
|
const client = http2.connect(`http://localhost:${port}`);
|
|
|
|
let remaining = 2;
|
|
function maybeClose() {
|
|
if (--remaining === 0) {
|
|
server.close();
|
|
client.destroy();
|
|
}
|
|
}
|
|
|
|
const req = client.request({ ':method': 'POST' });
|
|
req.on('response', common.mustCall());
|
|
req.resume();
|
|
req.on('end', common.mustCall(maybeClose));
|
|
const str = fs.createReadStream(loc);
|
|
str.on('end', common.mustCall(maybeClose));
|
|
str.pipe(req);
|
|
}));
|