mirror of
https://github.com/nodejs/node.git
synced 2025-05-09 19:16:58 +00:00
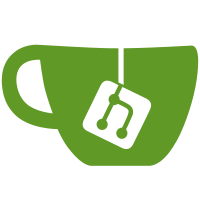
Handle edge case where stream pause is called between resume being called and actually evaluated. Other minor adjustments to avoid various edge cases around stream events. Add new tests that cover all changes. Fixes: https://github.com/nodejs/node/issues/15491 PR-URL: https://github.com/nodejs/node/pull/15503 Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
54 lines
1.4 KiB
JavaScript
54 lines
1.4 KiB
JavaScript
// Flags: --expose-http2
|
|
'use strict';
|
|
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
const assert = require('assert');
|
|
const h2 = require('http2');
|
|
|
|
// Check that pause & resume work as expected with Http2ServerRequest
|
|
|
|
const testStr = 'Request Body from Client';
|
|
|
|
const server = h2.createServer();
|
|
|
|
server.on('request', common.mustCall((req, res) => {
|
|
let data = '';
|
|
req.pause();
|
|
req.setEncoding('utf8');
|
|
req.on('data', common.mustCall((chunk) => (data += chunk)));
|
|
setTimeout(common.mustCall(() => {
|
|
assert.strictEqual(data, '');
|
|
req.resume();
|
|
}), common.platformTimeout(100));
|
|
req.on('end', common.mustCall(() => {
|
|
assert.strictEqual(data, testStr);
|
|
res.end();
|
|
}));
|
|
|
|
// shouldn't throw if underlying Http2Stream no longer exists
|
|
res.on('finish', common.mustCall(() => process.nextTick(() => {
|
|
assert.doesNotThrow(() => req.pause());
|
|
assert.doesNotThrow(() => req.resume());
|
|
})));
|
|
}));
|
|
|
|
server.listen(0, common.mustCall(() => {
|
|
const port = server.address().port;
|
|
|
|
const client = h2.connect(`http://localhost:${port}`);
|
|
const request = client.request({
|
|
':path': '/foobar',
|
|
':method': 'POST',
|
|
':scheme': 'http',
|
|
':authority': `localhost:${port}`
|
|
});
|
|
request.resume();
|
|
request.end(testStr);
|
|
request.on('end', common.mustCall(function() {
|
|
client.destroy();
|
|
server.close();
|
|
}));
|
|
}));
|