mirror of
https://github.com/nodejs/node.git
synced 2025-05-10 17:57:53 +00:00
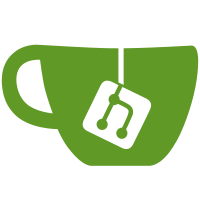
This patches changes the `safe_globals` internal module into a script that gets run during bootstrap and saves JavaScript builtins (primordials) into an object that is available for all other builtin modules to access lexically later. PR-URL: https://github.com/nodejs/node/pull/25816 Refs: https://github.com/nodejs/node/issues/18795 Reviewed-By: Bradley Farias <bradley.meck@gmail.com> Reviewed-By: Anna Henningsen <anna@addaleax.net> Reviewed-By: Gus Caplan <me@gus.host>
84 lines
2.0 KiB
JavaScript
84 lines
2.0 KiB
JavaScript
'use strict';
|
|
|
|
// This is only exposed for internal build steps and testing purposes.
|
|
// We create new copies of the source and the code cache
|
|
// so the resources eventually used to compile builtin modules
|
|
// cannot be tampered with even with --expose-internals.
|
|
|
|
const { NativeModule } = require('internal/bootstrap/loaders');
|
|
const {
|
|
getCodeCache, compileFunction
|
|
} = internalBinding('native_module');
|
|
const { hasTracing, hasInspector } = process.binding('config');
|
|
|
|
// Modules with source code compiled in js2c that
|
|
// cannot be compiled with the code cache.
|
|
const cannotBeRequired = [
|
|
'sys', // Deprecated.
|
|
'internal/v8_prof_polyfill',
|
|
'internal/v8_prof_processor',
|
|
|
|
'internal/per_context',
|
|
|
|
'internal/test/binding',
|
|
|
|
'internal/bootstrap/primordials',
|
|
'internal/bootstrap/loaders',
|
|
'internal/bootstrap/node'
|
|
];
|
|
|
|
// Skip modules that cannot be required when they are not
|
|
// built into the binary.
|
|
if (!hasInspector) {
|
|
cannotBeRequired.push(
|
|
'inspector',
|
|
'internal/util/inspector',
|
|
);
|
|
}
|
|
if (!hasTracing) {
|
|
cannotBeRequired.push('trace_events');
|
|
}
|
|
if (!process.versions.openssl) {
|
|
cannotBeRequired.push(
|
|
'crypto',
|
|
'https',
|
|
'http2',
|
|
'tls',
|
|
'_tls_common',
|
|
'_tls_wrap',
|
|
'internal/crypto/certificate',
|
|
'internal/crypto/cipher',
|
|
'internal/crypto/diffiehellman',
|
|
'internal/crypto/hash',
|
|
'internal/crypto/keygen',
|
|
'internal/crypto/keys',
|
|
'internal/crypto/pbkdf2',
|
|
'internal/crypto/random',
|
|
'internal/crypto/scrypt',
|
|
'internal/crypto/sig',
|
|
'internal/crypto/util',
|
|
'internal/http2/core',
|
|
'internal/http2/compat',
|
|
'internal/policy/manifest',
|
|
'internal/process/policy',
|
|
'internal/streams/lazy_transform',
|
|
);
|
|
}
|
|
|
|
const cachableBuiltins = [];
|
|
for (const id of NativeModule.map.keys()) {
|
|
if (id.startsWith('internal/deps') || id.startsWith('internal/main')) {
|
|
cannotBeRequired.push(id);
|
|
}
|
|
if (!cannotBeRequired.includes(id)) {
|
|
cachableBuiltins.push(id);
|
|
}
|
|
}
|
|
|
|
module.exports = {
|
|
cachableBuiltins,
|
|
getCodeCache,
|
|
compileFunction,
|
|
cannotBeRequired
|
|
};
|