mirror of
https://github.com/nodejs/node.git
synced 2025-05-17 10:27:12 +00:00
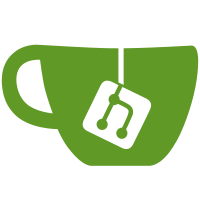
The breakOnSigint option follows different code paths, depending on the number of listeners for SIGINT. This commit updates the existing test to vary the number of SIGINT handlers. PR-URL: https://github.com/nodejs/node/pull/12512 Reviewed-By: David Cai <davidcai1993@yahoo.com> Reviewed-By: James M Snell <jasnell@gmail.com>
51 lines
1.4 KiB
JavaScript
51 lines
1.4 KiB
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const vm = require('vm');
|
|
|
|
const spawn = require('child_process').spawn;
|
|
|
|
if (common.isWindows) {
|
|
// No way to send CTRL_C_EVENT to processes from JS right now.
|
|
common.skip('platform not supported');
|
|
return;
|
|
}
|
|
|
|
if (process.argv[2] === 'child') {
|
|
const method = process.argv[3];
|
|
const listeners = +process.argv[4];
|
|
assert.ok(method);
|
|
assert.ok(typeof listeners, 'number');
|
|
|
|
const script = `process.send('${method}'); while(true) {}`;
|
|
const args = method === 'runInContext' ?
|
|
[vm.createContext({ process })] :
|
|
[];
|
|
const options = { breakOnSigint: true };
|
|
|
|
for (let i = 0; i < listeners; i++)
|
|
process.on('SIGINT', common.noop);
|
|
|
|
assert.throws(() => { vm[method](script, ...args, options); },
|
|
/^Error: Script execution interrupted\.$/);
|
|
return;
|
|
}
|
|
|
|
for (const method of ['runInThisContext', 'runInContext']) {
|
|
for (const listeners of [0, 1, 2]) {
|
|
const args = [__filename, 'child', method, listeners];
|
|
const child = spawn(process.execPath, args, {
|
|
stdio: [null, 'pipe', 'inherit', 'ipc']
|
|
});
|
|
|
|
child.on('message', common.mustCall(() => {
|
|
process.kill(child.pid, 'SIGINT');
|
|
}));
|
|
|
|
child.on('close', common.mustCall((code, signal) => {
|
|
assert.strictEqual(signal, null);
|
|
assert.strictEqual(code, 0);
|
|
}));
|
|
}
|
|
}
|