mirror of
https://github.com/nodejs/node.git
synced 2025-04-28 13:40:37 +00:00
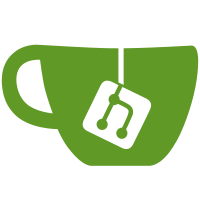
Move permission model from 1.1 (Active Development) to 2.0 (Stable). PR-URL: https://github.com/nodejs/node/pull/56201 Reviewed-By: Yagiz Nizipli <yagiz@nizipli.com> Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Santiago Gimeno <santiago.gimeno@gmail.com> Reviewed-By: Marco Ippolito <marcoippolito54@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Stephen Belanger <admin@stephenbelanger.com>
79 lines
2.1 KiB
JavaScript
79 lines
2.1 KiB
JavaScript
'use strict';
|
|
|
|
// This tests NODE_COMPILE_CACHE works in existing directory.
|
|
|
|
require('../common');
|
|
const { spawnSyncAndAssert } = require('../common/child_process');
|
|
const assert = require('assert');
|
|
const tmpdir = require('../common/tmpdir');
|
|
const fixtures = require('../common/fixtures');
|
|
const fs = require('fs');
|
|
|
|
function testAllowed(readDir, writeDir, envDir) {
|
|
console.log(readDir, writeDir, envDir); // Logging for debugging.
|
|
|
|
tmpdir.refresh();
|
|
const dummyDir = tmpdir.resolve('dummy');
|
|
fs.mkdirSync(dummyDir);
|
|
const script = tmpdir.resolve(dummyDir, 'empty.js');
|
|
fs.copyFileSync(fixtures.path('empty.js'), script);
|
|
// If the directory doesn't exist, permission will just be disallowed.
|
|
fs.mkdirSync(tmpdir.resolve(envDir));
|
|
|
|
spawnSyncAndAssert(
|
|
process.execPath,
|
|
[
|
|
'--permission',
|
|
`--allow-fs-read=${dummyDir}`,
|
|
`--allow-fs-read=${readDir}`,
|
|
`--allow-fs-write=${writeDir}`,
|
|
script,
|
|
],
|
|
{
|
|
env: {
|
|
...process.env,
|
|
NODE_DEBUG_NATIVE: 'COMPILE_CACHE',
|
|
NODE_COMPILE_CACHE: `${envDir}`
|
|
},
|
|
cwd: tmpdir.path
|
|
},
|
|
{
|
|
stderr(output) {
|
|
assert.match(output, /writing cache for .*empty\.js.*success/);
|
|
return true;
|
|
}
|
|
});
|
|
|
|
spawnSyncAndAssert(
|
|
process.execPath,
|
|
[
|
|
'--permission',
|
|
`--allow-fs-read=${dummyDir}`,
|
|
`--allow-fs-read=${readDir}`,
|
|
`--allow-fs-write=${writeDir}`,
|
|
script,
|
|
],
|
|
{
|
|
env: {
|
|
...process.env,
|
|
NODE_DEBUG_NATIVE: 'COMPILE_CACHE',
|
|
NODE_COMPILE_CACHE: `${envDir}`
|
|
},
|
|
cwd: tmpdir.path
|
|
},
|
|
{
|
|
stderr(output) {
|
|
assert.match(output, /cache for .*empty\.js was accepted/);
|
|
return true;
|
|
}
|
|
});
|
|
}
|
|
|
|
{
|
|
testAllowed(tmpdir.resolve('.compile_cache'), tmpdir.resolve('.compile_cache'), '.compile_cache');
|
|
testAllowed(tmpdir.resolve('.compile_cache'), tmpdir.resolve('.compile_cache'), tmpdir.resolve('.compile_cache'));
|
|
testAllowed('*', '*', '.compile_cache');
|
|
testAllowed('*', tmpdir.resolve('.compile_cache'), '.compile_cache');
|
|
testAllowed(tmpdir.resolve('.compile_cache'), '*', '.compile_cache');
|
|
}
|