mirror of
https://github.com/nodejs/node.git
synced 2025-05-10 19:17:56 +00:00
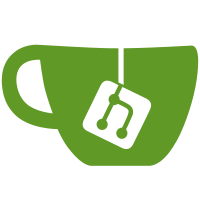
This patch refactors out a part of NativeModule.prototype.compile (in JS land) into a C++ NativeModule class, this enables a couple of possibilities: 1. By moving the code to the C++ land, we have more opportunity to specialize the compilation process of the native modules (e.g. compilation options, code cache) that is orthogonal to how user land modules are compiled 2. We can reuse the code to compile bootstrappers and context fixers and enable them to be compiled with the code cache later, since they are not loaded by NativeModule in the JS land their caching must be done in C++. 3. Since there is no need to pass the static data to JS for compilation anymore, this enables us to use (std::map<std::string, const char*>) in the generated node_code_cache.cc and node_javascript.cc later, and scope every actual access to the source of native modules to a std::map lookup instead of a lookup on a v8::Object in dictionary mode. This patch also refactor the code cache generator and tests a bit and trace the `withCodeCache` and `withoutCodeCache` in a Set instead of an Array, and makes sure that all the cachable builtins are tested. PR-URL: https://github.com/nodejs/node/pull/24221 Reviewed-By: Refael Ackermann <refack@gmail.com> Reviewed-By: Anna Henningsen <anna@addaleax.net>
78 lines
2.4 KiB
JavaScript
78 lines
2.4 KiB
JavaScript
'use strict';
|
|
|
|
// This is only exposed for internal build steps and testing purposes.
|
|
// We create new copies of the source and the code cache
|
|
// so the resources eventually used to compile builtin modules
|
|
// cannot be tampered with even with --expose-internals
|
|
|
|
const {
|
|
NativeModule
|
|
} = require('internal/bootstrap/loaders');
|
|
const {
|
|
source,
|
|
compileCodeCache
|
|
} = internalBinding('native_module');
|
|
const { hasTracing } = process.binding('config');
|
|
|
|
const depsModule = Object.keys(source).filter(
|
|
(key) => NativeModule.isDepsModule(key) || key.startsWith('internal/deps')
|
|
);
|
|
|
|
// Modules with source code compiled in js2c that
|
|
// cannot be compiled with the code cache
|
|
const cannotUseCache = [
|
|
'config',
|
|
'sys', // deprecated
|
|
'internal/v8_prof_polyfill',
|
|
'internal/v8_prof_processor',
|
|
|
|
'internal/per_context',
|
|
|
|
'internal/test/binding',
|
|
// TODO(joyeecheung): update the C++ side so that
|
|
// the code cache is also used when compiling these
|
|
// two files.
|
|
'internal/bootstrap/loaders',
|
|
'internal/bootstrap/node'
|
|
].concat(depsModule);
|
|
|
|
// Skip modules that cannot be required when they are not
|
|
// built into the binary.
|
|
if (process.config.variables.v8_enable_inspector !== 1) {
|
|
cannotUseCache.push('inspector');
|
|
cannotUseCache.push('internal/util/inspector');
|
|
}
|
|
if (!hasTracing) {
|
|
cannotUseCache.push('trace_events');
|
|
}
|
|
if (!process.versions.openssl) {
|
|
cannotUseCache.push('crypto');
|
|
cannotUseCache.push('https');
|
|
cannotUseCache.push('http2');
|
|
cannotUseCache.push('tls');
|
|
cannotUseCache.push('_tls_common');
|
|
cannotUseCache.push('_tls_wrap');
|
|
cannotUseCache.push('internal/crypto/certificate');
|
|
cannotUseCache.push('internal/crypto/cipher');
|
|
cannotUseCache.push('internal/crypto/diffiehellman');
|
|
cannotUseCache.push('internal/crypto/hash');
|
|
cannotUseCache.push('internal/crypto/keygen');
|
|
cannotUseCache.push('internal/crypto/pbkdf2');
|
|
cannotUseCache.push('internal/crypto/random');
|
|
cannotUseCache.push('internal/crypto/scrypt');
|
|
cannotUseCache.push('internal/crypto/sig');
|
|
cannotUseCache.push('internal/crypto/util');
|
|
cannotUseCache.push('internal/http2/core');
|
|
cannotUseCache.push('internal/http2/compat');
|
|
cannotUseCache.push('internal/streams/lazy_transform');
|
|
}
|
|
|
|
module.exports = {
|
|
cachableBuiltins: Object.keys(source).filter(
|
|
(key) => !cannotUseCache.includes(key)
|
|
),
|
|
getSource(id) { return source[id]; },
|
|
getCodeCache: compileCodeCache,
|
|
cannotUseCache
|
|
};
|