mirror of
https://github.com/nodejs/node.git
synced 2025-05-04 20:09:32 +00:00
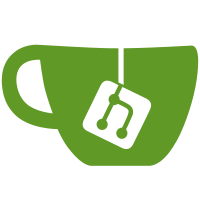
Fixes: https://github.com/nodejs/node/issues/38721 PR-URL: https://github.com/nodejs/node/pull/38723 Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de> Reviewed-By: Antoine du Hamel <duhamelantoine1995@gmail.com> Reviewed-By: Robert Nagy <ronagy@icloud.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: Zijian Liu <lxxyxzj@gmail.com> Reviewed-By: Darshan Sen <raisinten@gmail.com>
35 lines
802 B
JavaScript
35 lines
802 B
JavaScript
'use strict';
|
|
|
|
const {
|
|
SymbolAsyncIterator,
|
|
SymbolIterator,
|
|
} = primordials;
|
|
|
|
function isReadable(obj) {
|
|
return !!(obj && typeof obj.pipe === 'function' &&
|
|
typeof obj.on === 'function');
|
|
}
|
|
|
|
function isWritable(obj) {
|
|
return !!(obj && typeof obj.write === 'function' &&
|
|
typeof obj.on === 'function');
|
|
}
|
|
|
|
function isStream(obj) {
|
|
return isReadable(obj) || isWritable(obj);
|
|
}
|
|
|
|
function isIterable(obj, isAsync) {
|
|
if (obj == null) return false;
|
|
if (isAsync === true) return typeof obj[SymbolAsyncIterator] === 'function';
|
|
if (isAsync === false) return typeof obj[SymbolIterator] === 'function';
|
|
return typeof obj[SymbolAsyncIterator] === 'function' ||
|
|
typeof obj[SymbolIterator] === 'function';
|
|
}
|
|
|
|
module.exports = {
|
|
isIterable,
|
|
isReadable,
|
|
isStream,
|
|
};
|