mirror of
https://github.com/nodejs/node.git
synced 2025-05-08 12:49:23 +00:00
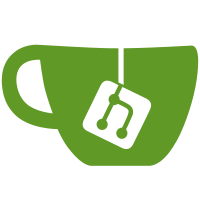
The current default is not ideal in most use cases. Therefore it is changed to showing unlimited depth in case util.inspect is called directly. The default is kept as before for console.log and similar. Using console.dir will now show a depth of up to five and console.assert / console.trace will show a unlimited depth. PR-URL: https://github.com/nodejs/node/pull/17907 Refs: https://github.com/nodejs/node/issues/12693 Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com>
36 lines
962 B
JavaScript
36 lines
962 B
JavaScript
// Flags: --expose_internals
|
|
'use strict';
|
|
require('../common');
|
|
const assert = require('assert');
|
|
const BufferList = require('internal/streams/BufferList');
|
|
const util = require('util');
|
|
|
|
// Test empty buffer list.
|
|
const emptyList = new BufferList();
|
|
|
|
emptyList.shift();
|
|
assert.deepStrictEqual(emptyList, new BufferList());
|
|
|
|
assert.strictEqual(emptyList.join(','), '');
|
|
|
|
assert.deepStrictEqual(emptyList.concat(0), Buffer.alloc(0));
|
|
|
|
// Test buffer list with one element.
|
|
const list = new BufferList();
|
|
list.push('foo');
|
|
|
|
assert.strictEqual(list.concat(1), 'foo');
|
|
|
|
assert.strictEqual(list.join(','), 'foo');
|
|
|
|
const shifted = list.shift();
|
|
assert.strictEqual(shifted, 'foo');
|
|
assert.deepStrictEqual(list, new BufferList());
|
|
|
|
const tmp = util.inspect.defaultOptions.colors;
|
|
util.inspect.defaultOptions = { colors: true };
|
|
assert.strictEqual(
|
|
util.inspect(list),
|
|
'BufferList { length: \u001b[33m0\u001b[39m }');
|
|
util.inspect.defaultOptions = { colors: tmp };
|