mirror of
https://github.com/nodejs/node.git
synced 2025-05-18 07:36:51 +00:00
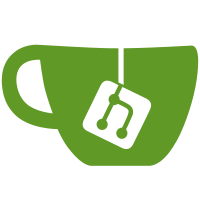
PR-URL: https://github.com/nodejs/node/pull/20190 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de> Reviewed-By: Tiancheng "Timothy" Gu <timothygu99@gmail.com> Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com>
72 lines
1.4 KiB
JavaScript
72 lines
1.4 KiB
JavaScript
'use strict'
|
|
|
|
/**
|
|
* response.js
|
|
*
|
|
* Response class provides content decoding
|
|
*/
|
|
|
|
const STATUS_CODES = require('http').STATUS_CODES
|
|
const Headers = require('./headers.js')
|
|
const Body = require('./body.js')
|
|
const clone = Body.clone
|
|
|
|
/**
|
|
* Response class
|
|
*
|
|
* @param Stream body Readable stream
|
|
* @param Object opts Response options
|
|
* @return Void
|
|
*/
|
|
class Response {
|
|
constructor (body, opts) {
|
|
if (!opts) opts = {}
|
|
Body.call(this, body, opts)
|
|
|
|
this.url = opts.url
|
|
this.status = opts.status || 200
|
|
this.statusText = opts.statusText || STATUS_CODES[this.status]
|
|
|
|
this.headers = new Headers(opts.headers)
|
|
|
|
Object.defineProperty(this, Symbol.toStringTag, {
|
|
value: 'Response',
|
|
writable: false,
|
|
enumerable: false,
|
|
configurable: true
|
|
})
|
|
}
|
|
|
|
/**
|
|
* Convenience property representing if the request ended normally
|
|
*/
|
|
get ok () {
|
|
return this.status >= 200 && this.status < 300
|
|
}
|
|
|
|
/**
|
|
* Clone this response
|
|
*
|
|
* @return Response
|
|
*/
|
|
clone () {
|
|
return new Response(clone(this), {
|
|
url: this.url,
|
|
status: this.status,
|
|
statusText: this.statusText,
|
|
headers: this.headers,
|
|
ok: this.ok
|
|
})
|
|
}
|
|
}
|
|
|
|
Body.mixIn(Response.prototype)
|
|
|
|
Object.defineProperty(Response.prototype, Symbol.toStringTag, {
|
|
value: 'ResponsePrototype',
|
|
writable: false,
|
|
enumerable: false,
|
|
configurable: true
|
|
})
|
|
module.exports = Response
|