mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 09:09:51 +00:00
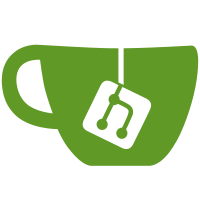
In the manual page, there is a statement that ciphersuites contain explicit default settings - all TLS 1.3 ciphersuites enabled. In node, we assume that an empty setting mean no ciphersuites and we disable TLS 1.3. A correct approach to disabling TLS 1.3 is to disable TLS 1.3 and by not override the default ciphersuits with an empty string. So, only override OpenSSL's TLS 1.3 ciphersuites with an explicit list of ciphers. If none are acceptable, the correct approach is to disable TLS 1.3 instead elsewhere. Fixes: https://github.com/nodejs/node/issues/43419 PR-URL: https://github.com/nodejs/node/pull/43427 Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Paolo Insogna <paolo@cowtech.it> Reviewed-By: James M Snell <jasnell@gmail.com>
92 lines
2.3 KiB
JavaScript
92 lines
2.3 KiB
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
|
|
const assert = require('assert');
|
|
const tls = require('tls');
|
|
const fixtures = require('../common/fixtures');
|
|
|
|
const key = fixtures.readKey('agent2-key.pem');
|
|
const cert = fixtures.readKey('agent2-cert.pem');
|
|
|
|
let nsuccess = 0;
|
|
let nerror = 0;
|
|
|
|
function loadDHParam(n) {
|
|
return fixtures.readKey(`dh${n}.pem`);
|
|
}
|
|
|
|
function test(size, err, next) {
|
|
const options = {
|
|
key: key,
|
|
cert: cert,
|
|
dhparam: loadDHParam(size),
|
|
ciphers: 'DHE-RSA-AES128-GCM-SHA256'
|
|
};
|
|
|
|
const server = tls.createServer(options, function(conn) {
|
|
conn.end();
|
|
});
|
|
|
|
server.on('close', function(isException) {
|
|
assert(!isException);
|
|
if (next) next();
|
|
});
|
|
|
|
server.listen(0, function() {
|
|
// Client set minimum DH parameter size to 2048 bits so that
|
|
// it fails when it make a connection to the tls server where
|
|
// dhparams is 1024 bits
|
|
const client = tls.connect({
|
|
minDHSize: 2048,
|
|
port: this.address().port,
|
|
rejectUnauthorized: false,
|
|
maxVersion: 'TLSv1.2',
|
|
}, function() {
|
|
nsuccess++;
|
|
server.close();
|
|
});
|
|
if (err) {
|
|
client.on('error', function(e) {
|
|
nerror++;
|
|
assert.strictEqual(e.code, 'ERR_TLS_DH_PARAM_SIZE');
|
|
server.close();
|
|
});
|
|
}
|
|
});
|
|
}
|
|
|
|
// A client connection fails with an error when a client has an
|
|
// 2048 bits minDHSize option and a server has 1024 bits dhparam
|
|
function testDHE1024() {
|
|
test(1024, true, testDHE2048);
|
|
}
|
|
|
|
// A client connection successes when a client has an
|
|
// 2048 bits minDHSize option and a server has 2048 bits dhparam
|
|
function testDHE2048() {
|
|
test(2048, false, null);
|
|
}
|
|
|
|
testDHE1024();
|
|
|
|
assert.throws(() => test(512, true, common.mustNotCall()),
|
|
/DH parameter is less than 1024 bits/);
|
|
|
|
let errMessage = /minDHSize is not a positive number/;
|
|
[0, -1, -Infinity, NaN].forEach((minDHSize) => {
|
|
assert.throws(() => tls.connect({ minDHSize }),
|
|
errMessage);
|
|
});
|
|
|
|
errMessage = /minDHSize is not a number/;
|
|
[true, false, null, undefined, {}, [], '', '1'].forEach((minDHSize) => {
|
|
assert.throws(() => tls.connect({ minDHSize }), errMessage);
|
|
});
|
|
|
|
process.on('exit', function() {
|
|
assert.strictEqual(nsuccess, 1);
|
|
assert.strictEqual(nerror, 1);
|
|
});
|