mirror of
https://github.com/nodejs/node.git
synced 2025-05-02 14:56:19 +00:00
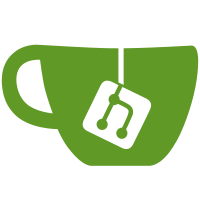
PR-URL: https://github.com/nodejs/node/pull/45712 Fixes: https://github.com/nodejs/node/issues/45648 Reviewed-By: Antoine du Hamel <duhamelantoine1995@gmail.com> Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com>
68 lines
2.0 KiB
JavaScript
68 lines
2.0 KiB
JavaScript
'use strict';
|
|
|
|
const {
|
|
ObjectCreate,
|
|
} = primordials;
|
|
const { getOptionValue } = require('internal/options');
|
|
const path = require('path');
|
|
const { shouldUseESMLoader } = require('internal/modules/utils');
|
|
|
|
function resolveMainPath(main) {
|
|
// Note extension resolution for the main entry point can be deprecated in a
|
|
// future major.
|
|
// Module._findPath is monkey-patchable here.
|
|
const { Module, toRealPath } = require('internal/modules/cjs/loader');
|
|
let mainPath = Module._findPath(path.resolve(main), null, true);
|
|
if (!mainPath)
|
|
return;
|
|
|
|
const preserveSymlinksMain = getOptionValue('--preserve-symlinks-main');
|
|
if (!preserveSymlinksMain)
|
|
mainPath = toRealPath(mainPath);
|
|
|
|
return mainPath;
|
|
}
|
|
|
|
function runMainESM(mainPath) {
|
|
const { loadESM } = require('internal/process/esm_loader');
|
|
const { pathToFileURL } = require('internal/url');
|
|
|
|
handleMainPromise(loadESM((esmLoader) => {
|
|
const main = path.isAbsolute(mainPath) ?
|
|
pathToFileURL(mainPath).href : mainPath;
|
|
return esmLoader.import(main, undefined, ObjectCreate(null));
|
|
}));
|
|
}
|
|
|
|
async function handleMainPromise(promise) {
|
|
const {
|
|
handleProcessExit,
|
|
} = require('internal/modules/esm/handle_process_exit');
|
|
process.on('exit', handleProcessExit);
|
|
try {
|
|
return await promise;
|
|
} finally {
|
|
process.off('exit', handleProcessExit);
|
|
}
|
|
}
|
|
|
|
// For backwards compatibility, we have to run a bunch of
|
|
// monkey-patchable code that belongs to the CJS loader (exposed by
|
|
// `require('module')`) even when the entry point is ESM.
|
|
function executeUserEntryPoint(main = process.argv[1]) {
|
|
const resolvedMain = resolveMainPath(main);
|
|
const useESMLoader = shouldUseESMLoader(resolvedMain);
|
|
if (useESMLoader) {
|
|
runMainESM(resolvedMain || main);
|
|
} else {
|
|
// Module._load is the monkey-patchable CJS module loader.
|
|
const { Module } = require('internal/modules/cjs/loader');
|
|
Module._load(main, null, true);
|
|
}
|
|
}
|
|
|
|
module.exports = {
|
|
executeUserEntryPoint,
|
|
handleMainPromise,
|
|
};
|