mirror of
https://github.com/nodejs/node.git
synced 2025-05-12 22:48:45 +00:00
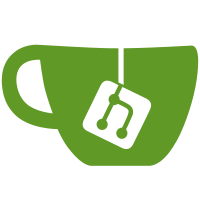
PR-URL: https://github.com/nodejs/node/pull/44119 Reviewed-By: Ruy Adorno <ruyadorno@google.com> Reviewed-By: Mohammed Keyvanzadeh <mohammadkeyvanzade94@gmail.com> Reviewed-By: Tobias Nießen <tniessen@tnie.de> Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: Myles Borins <myles.borins@gmail.com>
83 lines
1.8 KiB
JavaScript
83 lines
1.8 KiB
JavaScript
const chalk = require('chalk')
|
|
const ciDetect = require('@npmcli/ci-detect')
|
|
const runScript = require('@npmcli/run-script')
|
|
const readPackageJson = require('read-package-json-fast')
|
|
const npmlog = require('npmlog')
|
|
const log = require('proc-log')
|
|
const noTTY = require('./no-tty.js')
|
|
|
|
const nocolor = {
|
|
reset: s => s,
|
|
bold: s => s,
|
|
dim: s => s,
|
|
}
|
|
|
|
const run = async ({
|
|
args,
|
|
call,
|
|
color,
|
|
flatOptions,
|
|
locationMsg,
|
|
output = () => {},
|
|
path,
|
|
binPaths,
|
|
runPath,
|
|
scriptShell,
|
|
}) => {
|
|
// turn list of args into command string
|
|
const script = call || args.shift() || scriptShell
|
|
const colorize = color ? chalk : nocolor
|
|
|
|
// do the fakey runScript dance
|
|
// still should work if no package.json in cwd
|
|
const realPkg = await readPackageJson(`${path}/package.json`)
|
|
.catch(() => ({}))
|
|
const pkg = {
|
|
...realPkg,
|
|
scripts: {
|
|
...(realPkg.scripts || {}),
|
|
npx: script,
|
|
},
|
|
}
|
|
|
|
npmlog.disableProgress()
|
|
|
|
try {
|
|
if (script === scriptShell) {
|
|
const isTTY = !noTTY()
|
|
|
|
if (isTTY) {
|
|
if (ciDetect()) {
|
|
return log.warn('exec', 'Interactive mode disabled in CI environment')
|
|
}
|
|
|
|
locationMsg = locationMsg || ` at location:\n${colorize.dim(runPath)}`
|
|
|
|
output(`${
|
|
colorize.reset('\nEntering npm script environment')
|
|
}${
|
|
colorize.reset(locationMsg)
|
|
}${
|
|
colorize.bold('\nType \'exit\' or ^D when finished\n')
|
|
}`)
|
|
}
|
|
}
|
|
return await runScript({
|
|
...flatOptions,
|
|
pkg,
|
|
banner: false,
|
|
// we always run in cwd, not --prefix
|
|
path: runPath,
|
|
stdioString: true,
|
|
binPaths,
|
|
event: 'npx',
|
|
args,
|
|
stdio: 'inherit',
|
|
})
|
|
} finally {
|
|
npmlog.enableProgress()
|
|
}
|
|
}
|
|
|
|
module.exports = run
|