mirror of
https://github.com/nodejs/node.git
synced 2025-05-05 15:32:15 +00:00
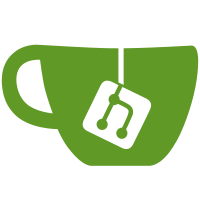
This commit adds tests for on(), once(), removeListener(), and prependOnceListener(), which all throw a TypeError if the listener argument is not a function. PR-URL: https://github.com/nodejs/node/pull/8168 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: Jeremiah Senkpiel <fishrock123@rocketmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
37 lines
780 B
JavaScript
37 lines
780 B
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const EventEmitter = require('events');
|
|
|
|
const e = new EventEmitter();
|
|
|
|
e.once('hello', common.mustCall(function(a, b) {}));
|
|
|
|
e.emit('hello', 'a', 'b');
|
|
e.emit('hello', 'a', 'b');
|
|
e.emit('hello', 'a', 'b');
|
|
e.emit('hello', 'a', 'b');
|
|
|
|
var remove = function() {
|
|
common.fail('once->foo should not be emitted');
|
|
};
|
|
|
|
e.once('foo', remove);
|
|
e.removeListener('foo', remove);
|
|
e.emit('foo');
|
|
|
|
e.once('e', common.mustCall(function() {
|
|
e.emit('e');
|
|
}));
|
|
|
|
e.once('e', common.mustCall(function() {}));
|
|
|
|
e.emit('e');
|
|
|
|
// Verify that the listener must be a function
|
|
assert.throws(() => {
|
|
const ee = new EventEmitter();
|
|
|
|
ee.once('foo', null);
|
|
}, /^TypeError: "listener" argument must be a function$/);
|