mirror of
https://github.com/nodejs/node.git
synced 2025-05-12 02:02:29 +00:00
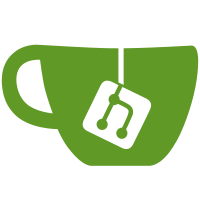
This commit sets the spawnSync() exit code to null when the child is killed via signal. This brings the behavior more in sync with spawn(). Fixes: https://github.com/nodejs/node/issues/11284 PR-URL: https://github.com/nodejs/node/pull/11288 Reviewed-By: Santiago Gimeno <santiago.gimeno@gmail.com> Reviewed-By: Anna Henningsen <anna@addaleax.net> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Evan Lucas <evanlucas@me.com>
51 lines
1.4 KiB
JavaScript
51 lines
1.4 KiB
JavaScript
'use strict';
|
|
require('../common');
|
|
const assert = require('assert');
|
|
const cp = require('child_process');
|
|
|
|
if (process.argv[2] === 'child') {
|
|
setInterval(() => {}, 1000);
|
|
} else {
|
|
const { SIGKILL } = process.binding('constants').os.signals;
|
|
|
|
function spawn(killSignal) {
|
|
const child = cp.spawnSync(process.execPath,
|
|
[__filename, 'child'],
|
|
{killSignal, timeout: 100});
|
|
|
|
assert.strictEqual(child.status, null);
|
|
assert.strictEqual(child.error.code, 'ETIMEDOUT');
|
|
return child;
|
|
}
|
|
|
|
// Verify that an error is thrown for unknown signals.
|
|
assert.throws(() => {
|
|
spawn('SIG_NOT_A_REAL_SIGNAL');
|
|
}, /Error: Unknown signal: SIG_NOT_A_REAL_SIGNAL/);
|
|
|
|
// Verify that the default kill signal is SIGTERM.
|
|
{
|
|
const child = spawn();
|
|
|
|
assert.strictEqual(child.signal, 'SIGTERM');
|
|
assert.strictEqual(child.options.killSignal, undefined);
|
|
}
|
|
|
|
// Verify that a string signal name is handled properly.
|
|
{
|
|
const child = spawn('SIGKILL');
|
|
|
|
assert.strictEqual(child.signal, 'SIGKILL');
|
|
assert.strictEqual(child.options.killSignal, SIGKILL);
|
|
}
|
|
|
|
// Verify that a numeric signal is handled properly.
|
|
{
|
|
const child = spawn(SIGKILL);
|
|
|
|
assert.strictEqual(typeof SIGKILL, 'number');
|
|
assert.strictEqual(child.signal, 'SIGKILL');
|
|
assert.strictEqual(child.options.killSignal, SIGKILL);
|
|
}
|
|
}
|