mirror of
https://github.com/nodejs/node.git
synced 2025-05-05 01:22:05 +00:00
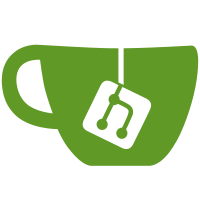
Ref: https://github.com/nodejs/node-convergence-archive/issues/13 This adds a new check for header and trailer fields names and method names to ensure that they conform to the HTTP token rule. If they do not, a `TypeError` is thrown. Previously this had an additional `strictMode` option that has been removed in favor of making the strict check the default (and only) behavior. Doc and test case are included. On the client-side ```javascript var http = require('http'); var url = require('url'); var p = url.parse('http://localhost:8888'); p.headers = {'testing 123': 123}; http.client(p, function(res) { }); // throws ``` On the server-side ```javascript var http = require('http'); var server = http.createServer(function(req,res) { res.setHeader('testing 123', 123); // throws res.end('...'); }); ``` Reviewed-By: Sakthipriyan Vairamani <thechargingvolcano@gmail.com> Reviewed-By: Trevor Norris <trevnorris@nodejs.org> Reviewed-By: Сковорода Никита Андреевич <chalkerx@gmail.com> Reviewed-By: Rod Vagg <rod@vagg.org> PR-URL: https://github.com/nodejs/node/pull/2526
57 lines
1.1 KiB
JavaScript
57 lines
1.1 KiB
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const EventEmitter = require('events');
|
|
const http = require('http');
|
|
|
|
const ee = new EventEmitter();
|
|
var count = 3;
|
|
|
|
const server = http.createServer(function(req, res) {
|
|
assert.doesNotThrow(function() {
|
|
res.setHeader('testing_123', 123);
|
|
});
|
|
assert.throws(function() {
|
|
res.setHeader('testing 123', 123);
|
|
}, TypeError);
|
|
res.end('');
|
|
});
|
|
server.listen(common.PORT, function() {
|
|
|
|
http.get({port: common.PORT}, function() {
|
|
ee.emit('done');
|
|
});
|
|
|
|
assert.throws(
|
|
function() {
|
|
var options = {
|
|
port: common.PORT,
|
|
headers: {'testing 123': 123}
|
|
};
|
|
http.get(options, function() {});
|
|
},
|
|
function(err) {
|
|
ee.emit('done');
|
|
if (err instanceof TypeError) return true;
|
|
}
|
|
);
|
|
|
|
assert.doesNotThrow(
|
|
function() {
|
|
var options = {
|
|
port: common.PORT,
|
|
headers: {'testing_123': 123}
|
|
};
|
|
http.get(options, function() {
|
|
ee.emit('done');
|
|
});
|
|
}, TypeError
|
|
);
|
|
});
|
|
|
|
ee.on('done', function() {
|
|
if (--count === 0) {
|
|
server.close();
|
|
}
|
|
});
|