mirror of
https://github.com/nodejs/node.git
synced 2025-05-01 17:03:34 +00:00
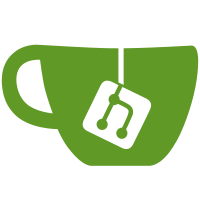
This changes the error handling model of ServerHttp2Stream, ServerHttp2Request and ServerHttp2Response. An 'error' emitted on ServerHttp2Stream will not go to 'uncaughtException' anymore, but to the server 'streamError'. On the stream 'error', ServerHttp2Request will emit 'abort', while ServerHttp2Response would do nothing See: https://github.com/nodejs/node/issues/14963 PR-URL: https://github.com/nodejs/node/pull/14991 Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Benjamin Gruenbaum <benjamingr@gmail.com>
57 lines
1.6 KiB
JavaScript
57 lines
1.6 KiB
JavaScript
// Flags: --expose-http2
|
|
'use strict';
|
|
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
const assert = require('assert');
|
|
const h2 = require('http2');
|
|
const NGHTTP2_INTERNAL_ERROR = h2.constants.NGHTTP2_INTERNAL_ERROR;
|
|
|
|
const server = h2.createServer();
|
|
|
|
// Do not mustCall the server side callbacks, they may or may not be called
|
|
// depending on the OS. The determination is based largely on operating
|
|
// system specific timings
|
|
server.on('stream', (stream) => {
|
|
// Do not wrap in a must call or use common.expectsError (which now uses
|
|
// must call). The error may or may not be reported depending on operating
|
|
// system specific timings.
|
|
stream.on('error', (err) => {
|
|
assert.strictEqual(err.code, 'ERR_HTTP2_STREAM_ERROR');
|
|
assert.strictEqual(err.message, 'Stream closed with error code 2');
|
|
});
|
|
stream.respond({});
|
|
stream.end();
|
|
});
|
|
|
|
server.listen(0);
|
|
|
|
server.on('listening', common.mustCall(() => {
|
|
|
|
const client = h2.connect(`http://localhost:${server.address().port}`);
|
|
|
|
const req = client.request({ ':path': '/' });
|
|
const err = new Error('test');
|
|
req.destroy(err);
|
|
|
|
req.on('error', common.mustCall((err) => {
|
|
common.expectsError({
|
|
type: Error,
|
|
message: 'test'
|
|
})(err);
|
|
}));
|
|
|
|
req.on('streamClosed', common.mustCall((code) => {
|
|
assert.strictEqual(req.rstCode, NGHTTP2_INTERNAL_ERROR);
|
|
assert.strictEqual(code, NGHTTP2_INTERNAL_ERROR);
|
|
server.close();
|
|
client.destroy();
|
|
}));
|
|
|
|
req.on('response', common.mustNotCall());
|
|
req.resume();
|
|
req.on('end', common.mustCall());
|
|
|
|
}));
|