mirror of
https://github.com/nodejs/node.git
synced 2025-04-30 23:56:58 +00:00
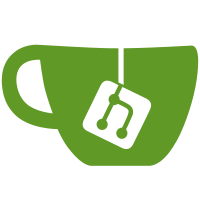
The copyright and license notice is already in the LICENSE file. There is no justifiable reason to also require that it be included in every file, since the individual files are not individually distributed except as part of the entire package.
49 lines
1.7 KiB
JavaScript
49 lines
1.7 KiB
JavaScript
(function() {
|
|
var assert = require('assert'),
|
|
child = require('child_process'),
|
|
util = require('util'),
|
|
common = require('../common');
|
|
if (process.env['TEST_INIT']) {
|
|
util.print('Loaded successfully!');
|
|
} else {
|
|
// change CWD as we do this test so its not dependant on current CWD
|
|
// being in the test folder
|
|
process.chdir(__dirname);
|
|
|
|
// slow but simple
|
|
var envCopy = JSON.parse(JSON.stringify(process.env));
|
|
envCopy.TEST_INIT = 1;
|
|
|
|
child.exec(process.execPath + ' test-init', {env: envCopy},
|
|
function(err, stdout, stderr) {
|
|
assert.equal(stdout, 'Loaded successfully!',
|
|
'`node test-init` failed!');
|
|
});
|
|
child.exec(process.execPath + ' test-init.js', {env: envCopy},
|
|
function(err, stdout, stderr) {
|
|
assert.equal(stdout, 'Loaded successfully!',
|
|
'`node test-init.js` failed!');
|
|
});
|
|
|
|
// test-init-index is in fixtures dir as requested by ry, so go there
|
|
process.chdir(common.fixturesDir);
|
|
|
|
child.exec(process.execPath + ' test-init-index', {env: envCopy},
|
|
function(err, stdout, stderr) {
|
|
assert.equal(stdout, 'Loaded successfully!',
|
|
'`node test-init-index failed!');
|
|
});
|
|
|
|
// ensures that `node fs` does not mistakenly load the native 'fs' module
|
|
// instead of the desired file and that the fs module loads as
|
|
// expected in node
|
|
process.chdir(common.fixturesDir + '/test-init-native/');
|
|
|
|
child.exec(process.execPath + ' fs', {env: envCopy},
|
|
function(err, stdout, stderr) {
|
|
assert.equal(stdout, 'fs loaded successfully',
|
|
'`node fs` failed!');
|
|
});
|
|
}
|
|
})();
|